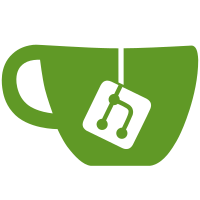
18 changed files with 3027 additions and 709 deletions
@ -0,0 +1,27 @@
@@ -0,0 +1,27 @@
|
||||
Copyright (c) 2012-2014, Olaf van Zandwijk |
||||
All rights reserved. |
||||
|
||||
Redistribution and use in source and binary forms, with or without modification, |
||||
are permitted provided that the following conditions are met: |
||||
|
||||
1. Redistributions of source code must retain the above copyright notice, |
||||
this list of conditions and the following disclaimer. |
||||
|
||||
2. Redistributions in binary form must reproduce the above copyright notice, |
||||
this list of conditions and the following disclaimer in the documentation |
||||
and/or other materials provided with the distribution. |
||||
|
||||
3. Neither the name of the copyright holder nor the names of its contributors |
||||
may be used to endorse or promote products derived from this software without |
||||
specific prior written permission. |
||||
|
||||
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND |
||||
ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED |
||||
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE |
||||
DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR |
||||
ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES |
||||
(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; |
||||
LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON |
||||
ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT |
||||
(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS |
||||
SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. |
@ -0,0 +1,89 @@
@@ -0,0 +1,89 @@
|
||||
#!/bin/bash |
||||
# |
||||
# Script to make a proxy (ie HAProxy) capable of monitoring Galera cluster nodes properly |
||||
# |
||||
# Author: Olaf van Zandwijk <olaf.vanzandwijk@nedap.com> |
||||
# Author: Raghavendra Prabhu <raghavendra.prabhu@percona.com> |
||||
# Author: Ryan O'Hara <rohara@redhat.com> |
||||
# |
||||
# Documentation and download: https://github.com/olafz/percona-clustercheck |
||||
# |
||||
# Based on the original script from Unai Rodriguez |
||||
# |
||||
|
||||
if [ -f @INSTALL_SYSCONFDIR@/sysconfig/clustercheck ]; then |
||||
. @INSTALL_SYSCONFDIR@/sysconfig/clustercheck |
||||
fi |
||||
|
||||
MYSQL_USERNAME="${MYSQL_USERNAME-clustercheckuser}" |
||||
MYSQL_PASSWORD="${MYSQL_PASSWORD-clustercheckpassword!}" |
||||
MYSQL_HOST="${MYSQL_HOST:-127.0.0.1}" |
||||
MYSQL_PORT="${MYSQL_PORT:-3306}" |
||||
ERR_FILE="${ERR_FILE:-/dev/null}" |
||||
AVAILABLE_WHEN_DONOR=${AVAILABLE_WHEN_DONOR:-0} |
||||
AVAILABLE_WHEN_READONLY=${AVAILABLE_WHEN_READONLY:-1} |
||||
DEFAULTS_EXTRA_FILE=${DEFAULTS_EXTRA_FILE:-@INSTALL_SYSCONFDIR@/my.cnf} |
||||
|
||||
#Timeout exists for instances where mysqld may be hung |
||||
TIMEOUT=10 |
||||
|
||||
if [[ -r $DEFAULTS_EXTRA_FILE ]];then |
||||
MYSQL_CMDLINE="mysql --defaults-extra-file=$DEFAULTS_EXTRA_FILE -nNE \ |
||||
--connect-timeout=$TIMEOUT \ |
||||
--user=${MYSQL_USERNAME} --password=${MYSQL_PASSWORD} \ |
||||
--host=${MYSQL_HOST} --port=${MYSQL_PORT}" |
||||
else |
||||
MYSQL_CMDLINE="mysql -nNE --connect-timeout=$TIMEOUT \ |
||||
--user=${MYSQL_USERNAME} --password=${MYSQL_PASSWORD} \ |
||||
--host=${MYSQL_HOST} --port=${MYSQL_PORT}" |
||||
fi |
||||
# |
||||
# Perform the query to check the wsrep_local_state |
||||
# |
||||
WSREP_STATUS=$($MYSQL_CMDLINE -e "SHOW STATUS LIKE 'wsrep_local_state';" \ |
||||
2>${ERR_FILE} | tail -1 2>>${ERR_FILE}) |
||||
|
||||
if [[ "${WSREP_STATUS}" == "4" ]] || [[ "${WSREP_STATUS}" == "2" && ${AVAILABLE_WHEN_DONOR} == 1 ]] |
||||
then |
||||
# Check only when set to 0 to avoid latency in response. |
||||
if [[ $AVAILABLE_WHEN_READONLY -eq 0 ]];then |
||||
READ_ONLY=$($MYSQL_CMDLINE -e "SHOW GLOBAL VARIABLES LIKE 'read_only';" \ |
||||
2>${ERR_FILE} | tail -1 2>>${ERR_FILE}) |
||||
|
||||
if [[ "${READ_ONLY}" == "ON" ]];then |
||||
# Galera cluster node local state is 'Synced', but it is in |
||||
# read-only mode. The variable AVAILABLE_WHEN_READONLY is set to 0. |
||||
# => return HTTP 503 |
||||
# Shell return-code is 1 |
||||
echo -en "HTTP/1.1 503 Service Unavailable\r\n" |
||||
echo -en "Content-Type: text/plain\r\n" |
||||
echo -en "Connection: close\r\n" |
||||
echo -en "Content-Length: 35\r\n" |
||||
echo -en "\r\n" |
||||
echo -en "Galera cluster node is read-only.\r\n" |
||||
sleep 0.1 |
||||
exit 1 |
||||
fi |
||||
fi |
||||
# Galera cluster node local state is 'Synced' => return HTTP 200 |
||||
# Shell return-code is 0 |
||||
echo -en "HTTP/1.1 200 OK\r\n" |
||||
echo -en "Content-Type: text/plain\r\n" |
||||
echo -en "Connection: close\r\n" |
||||
echo -en "Content-Length: 32\r\n" |
||||
echo -en "\r\n" |
||||
echo -en "Galera cluster node is synced.\r\n" |
||||
sleep 0.1 |
||||
exit 0 |
||||
else |
||||
# Galera cluster node local state is not 'Synced' => return HTTP 503 |
||||
# Shell return-code is 1 |
||||
echo -en "HTTP/1.1 503 Service Unavailable\r\n" |
||||
echo -en "Content-Type: text/plain\r\n" |
||||
echo -en "Connection: close\r\n" |
||||
echo -en "Content-Length: 36\r\n" |
||||
echo -en "\r\n" |
||||
echo -en "Galera cluster node is not synced.\r\n" |
||||
sleep 0.1 |
||||
exit 1 |
||||
fi |
@ -0,0 +1,29 @@
@@ -0,0 +1,29 @@
|
||||
This scirpt is ran by the systemd service. |
||||
In Fedora the service has priviledges dropped to the mysql user. |
||||
Thus "chown 0" will always fail |
||||
|
||||
Never parse 'ls' output! |
||||
http://mywiki.wooledge.org/BashFAQ/087 |
||||
|
||||
--- mariadb-10.4.7/scripts/mysql_install_db.sh 2019-07-30 13:32:16.000000000 +0200 |
||||
+++ mariadb-10.4.7/scripts/mysql_install_db.sh_patched 2019-08-22 16:29:28.341484925 +0200 |
||||
@@ -490,13 +490,16 @@ then |
||||
fi |
||||
if test -z "$srcdir" |
||||
then |
||||
- chown 0 "$pamtooldir/auth_pam_tool_dir/auth_pam_tool" && \ |
||||
- chmod 04755 "$pamtooldir/auth_pam_tool_dir/auth_pam_tool" |
||||
- if test $? -ne 0 |
||||
+ if [ `stat "$pamtooldir/auth_pam_tool_dir/auth_pam_tool" -c %u` -ne 0 ] |
||||
then |
||||
+ chown 0 "$pamtooldir/auth_pam_tool_dir/auth_pam_tool" && \ |
||||
+ chmod 04755 "$pamtooldir/auth_pam_tool_dir/auth_pam_tool" |
||||
+ if test $? -ne 0 |
||||
+ then |
||||
echo "Couldn't set an owner to '$pamtooldir/auth_pam_tool_dir/auth_pam_tool'." |
||||
echo " It must be root, the PAM authentication plugin doesn't work otherwise.." |
||||
echo |
||||
+ fi |
||||
fi |
||||
fi |
||||
args="$args --user=$user" |
@ -0,0 +1,27 @@
@@ -0,0 +1,27 @@
|
||||
Upstream PR: https://github.com/MariaDB/server/pull/1081 |
||||
|
||||
From d2cbf56d36e422802aa7e53ec0f4e6be8fd53cf5 Mon Sep 17 00:00:00 2001 |
||||
From: Honza Horak <hhorak@redhat.com> |
||||
Date: Wed, 9 Jan 2019 20:17:29 +0100 |
||||
Subject: [PATCH] Make the PYTHON_SHEBANG value configurable |
||||
|
||||
In Fedora 30 it is required to specify either /usr/bin/python2 or /usr/bin/python3 in the shebang, so we need a way to say explicit shebang, ideally in the cmake call. |
||||
--- |
||||
CMakeLists.txt | 4 +++- |
||||
1 file changed, 3 insertions(+), 1 deletion(-) |
||||
|
||||
diff --git a/CMakeLists.txt b/CMakeLists.txt |
||||
index a139c9e5fa4..ccccb08bef1 100644 |
||||
--- a/CMakeLists.txt |
||||
+++ b/CMakeLists.txt |
||||
@@ -342,7 +342,9 @@ MYSQL_CHECK_SSL() |
||||
MYSQL_CHECK_READLINE() |
||||
|
||||
SET(MALLOC_LIBRARY "system") |
||||
-SET(PYTHON_SHEBANG "/usr/bin/env python" CACHE STRING "python shebang") |
||||
+IF(NOT DEFINED PYTHON_SHEBANG) |
||||
+ SET(PYTHON_SHEBANG "/usr/bin/env python") |
||||
+ENDIF() |
||||
MARK_AS_ADVANCED(PYTHON_SHEBANG) |
||||
|
||||
CHECK_PCRE() |
File diff suppressed because it is too large
Load Diff
@ -0,0 +1,52 @@
@@ -0,0 +1,52 @@
|
||||
diff -up mariadb-10.3.9/mysql-test/main/ssl_cipher.test.fixtest mariadb-10.3.9/mysql-test/main/ssl_cipher.test |
||||
--- mariadb-10.3.9/mysql-test/main/ssl_cipher.test.fixtest 2019-01-27 19:39:19.610027153 +0100 |
||||
+++ mariadb-10.3.9/mysql-test/main/ssl_cipher.test 2019-01-27 19:42:10.045430776 +0100 |
||||
@@ -13,7 +13,9 @@ |
||||
connect (ssl_con,localhost,root,,,,,SSL); |
||||
|
||||
# Check Cipher Name and Cipher List |
||||
+--replace_regex /TLS_AES_.*/AES128-SHA/ |
||||
SHOW STATUS LIKE 'Ssl_cipher'; |
||||
+--replace_regex /TLS_AES_.*/AES128-SHA/ |
||||
SHOW STATUS LIKE 'Ssl_cipher_list'; |
||||
|
||||
connection default; |
||||
diff -up mariadb-10.3.9/mysql-test/main/ssl.result.fixtestssl mariadb-10.3.9/mysql-test/main/ssl.result |
||||
--- mariadb-10.3.9/mysql-test/main/ssl.result.fixtestssl 2019-01-27 20:41:52.605213547 +0100 |
||||
+++ mariadb-10.3.9/mysql-test/main/ssl.result 2019-01-27 20:42:03.977320005 +0100 |
||||
@@ -2176,7 +2176,7 @@ still connected? |
||||
connection default; |
||||
disconnect ssl_con; |
||||
create user mysqltest_1@localhost; |
||||
-grant usage on mysqltest.* to mysqltest_1@localhost require cipher "AES256-SHA"; |
||||
+grant usage on mysqltest.* to mysqltest_1@localhost require cipher "TLS_AES_256_GCM_SHA384"; |
||||
Variable_name Value |
||||
-Ssl_cipher AES256-SHA |
||||
+Ssl_cipher TLS_AES_256_GCM_SHA384 |
||||
drop user mysqltest_1@localhost; |
||||
diff -up mariadb-10.3.9/mysql-test/main/ssl.test.fixtestssl mariadb-10.3.9/mysql-test/main/ssl.test |
||||
--- mariadb-10.3.9/mysql-test/main/ssl.test.fixtestssl 2019-01-27 20:40:39.756531579 +0100 |
||||
+++ mariadb-10.3.9/mysql-test/main/ssl.test 2019-01-27 20:41:02.631745724 +0100 |
||||
@@ -33,8 +33,8 @@ connection default; |
||||
disconnect ssl_con; |
||||
|
||||
create user mysqltest_1@localhost; |
||||
-grant usage on mysqltest.* to mysqltest_1@localhost require cipher "AES256-SHA"; |
||||
---exec $MYSQL -umysqltest_1 --ssl-cipher=AES256-SHA -e "show status like 'ssl_cipher'" 2>&1 |
||||
+grant usage on mysqltest.* to mysqltest_1@localhost require cipher "TLS_AES_256_GCM_SHA384"; |
||||
+--exec $MYSQL -umysqltest_1 --ssl-cipher=TLS_AES_256_GCM_SHA384 -e "show status like 'ssl_cipher'" 2>&1 |
||||
drop user mysqltest_1@localhost; |
||||
|
||||
# Wait till all disconnects are completed |
||||
diff -up mariadb-10.3.9/mysql-test/main/ssl_cert_verify.test.fixcerttest mariadb-10.3.9/mysql-test/main/ssl_cert_verify.test |
||||
--- mariadb-10.3.9/mysql-test/main/ssl_cert_verify.test.fixcerttest 2019-01-27 21:11:12.280726041 +0100 |
||||
+++ mariadb-10.3.9/mysql-test/main/ssl_cert_verify.test 2019-01-27 21:10:01.034041434 +0100 |
||||
@@ -30,7 +30,7 @@ let $ssl_verify_pass_path = --ssl --ssl- |
||||
--enable_reconnect |
||||
--source include/wait_until_connected_again.inc |
||||
|
||||
---replace_result TLSv1.2 TLS_VERSION TLSv1.1 TLS_VERSION TLSv1 TLS_VERSION |
||||
+--replace_result TLSv1.3 TLS_VERSION TLSv1.2 TLS_VERSION TLSv1.1 TLS_VERSION TLSv1 TLS_VERSION |
||||
--exec $MYSQL --protocol=tcp --ssl-ca=$MYSQL_TEST_DIR/std_data/ca-cert-verify.pem --ssl-verify-server-cert -e "SHOW STATUS like 'Ssl_version'" |
||||
|
||||
--echo # restart server using restart |
@ -1,12 +1,37 @@
@@ -1,12 +1,37 @@
|
||||
# Fails since 10.3.8, only on armv7hl |
||||
versioning.auto_increment : |
||||
versioning.commit_id : |
||||
versioning.delete : |
||||
versioning.foreign : |
||||
versioning.insert : |
||||
versioning.insert2 : |
||||
versioning.select2 : |
||||
versioning.update : |
||||
|
||||
connect.bin : rhbz#1096787 (pass on aarch64) |
||||
connect.endian : rhbz#1096787 |
||||
|
||||
main.partition_exchange : rhbz#1096787 |
||||
main.analyze_stmt_orderby : rhbz#1096787 |
||||
main.explain_json_innodb : rhbz#1096787 |
||||
main.explain_json_format_partitions : rhbz#1096787 |
||||
main.analyze_format_json : rhbz#1096787 |
||||
main.explain_json : rhbz#1096787 |
||||
main.subselect_cache : rhbz#1096787 |
||||
main.type_year : rhbz#1096787 |
||||
# Fails since 10.3.8, on both armv7hl and aarch64 |
||||
mariabackup.system_versioning : |
||||
|
||||
# Fails since 10.3.9, on both armv7hl and aarch64 |
||||
disks.disks : |
||||
encryption.create_or_replace : |
||||
federated.federatedx_versioning : |
||||
|
||||
# Fails since 10.3.9, only on armv7hl |
||||
versioning.alter : |
||||
versioning.replace : |
||||
versioning.select : |
||||
versioning.truncate : |
||||
versioning.trx_id : |
||||
|
||||
# Fails since 10.3.9, only on aarch64 |
||||
innodb_gis.kill_server : |
||||
innodb_gis.rtree_search : |
||||
innodb.innodb_defrag_concurrent : |
||||
parts.partition_alter1_1_2_innodb : |
||||
parts.partition_alter1_2_innodb : |
||||
parts.partition_alter2_1_1_innodb : |
||||
parts.partition_alter2_1_2_innodb : |
||||
parts.partition_alter2_2_1_innodb : |
||||
parts.partition_alter2_2_2_innodb : |
||||
parts.partition_basic_innodb : |
||||
parts.part_supported_sql_func_innodb : |
||||
|
@ -1,14 +1,63 @@
@@ -1,14 +1,63 @@
|
||||
# These tests fail with MariaDB 10: |
||||
|
||||
main.userstat : rhbz#1096787 |
||||
main.set_statement_notembedded_binlog : rhbz#1096787 |
||||
main.ssl_7937 : rhbz#1096787 |
||||
main.ssl_crl_clients : rhbz#1096787 |
||||
main.openssl_1 : rhbz#1096787 |
||||
main.ssl : rhbz#1096787 |
||||
main.ssl_8k_key : rhbz#1096787 |
||||
main.ssl_compress : rhbz#1096787 |
||||
main.ssl_timeout : rhbz#1096787 |
||||
perfschema.nesting : rhbz#1096787 |
||||
perfschema.socket_summary_by_event_name_func : rhbz#1096787 |
||||
perfschema.socket_summary_by_instance_func : rhbz#1096787 |
||||
# The SSL test are failing correctly. Fro more explanation, see: |
||||
# https://jira.mariadb.org/browse/MDEV-8404?focusedCommentId=84275&page=com.atlassian.jira.plugin.system.issuetabpanels%3Acomment-tabpanel#comment-84275 |
||||
main.ssl_7937 : #1399847 |
||||
main.ssl_crl_clients : #1399847 |
||||
main.ssl_8k_key : |
||||
|
||||
# |
||||
perfschema.nesting : #1399847 |
||||
perfschema.socket_summary_by_instance_func : #1399847 |
||||
perfschema.socket_summary_by_event_name_func : |
||||
|
||||
# ------------------------------ |
||||
# Tests that fails because of 'Self Signed Certificate in the Certificate Chain' |
||||
perfschema.cnf_option : |
||||
|
||||
rpl.rpl_row_img_blobs : |
||||
rpl.rpl_row_img_eng_min : |
||||
rpl.rpl_row_img_eng_noblob : |
||||
|
||||
sys_vars.slave_parallel_threads_basic : |
||||
|
||||
# ------------------------------ |
||||
# Fails since 10.1.12 |
||||
innodb.innodb_defrag_binlog : |
||||
|
||||
# on x86_64 after 10.2.12 and 10.2.13 after some unidentified change in Rawhide buildroot |
||||
mroonga/storage.index_multiple_column_range_all_used_less_than : |
||||
mroonga/storage.index_multiple_column_range_all_used_less_than_or_equal : |
||||
mroonga/storage.index_multiple_column_range_partially_used_have_prefix_less_than : |
||||
mroonga/storage.index_multiple_column_range_partially_used_have_prefix_less_than_or_equal : |
||||
mroonga/storage.index_multiple_column_range_partially_used_no_prefix_less_than : |
||||
mroonga/storage.index_multiple_column_range_partially_used_no_prefix_less_than_or_equal : |
||||
mroonga/storage.optimization_order_limit_optimized_datetime_less_than : |
||||
mroonga/storage.optimization_order_limit_optimized_datetime_less_than_or_equal : |
||||
|
||||
# Fails everywhere since 10.2.15 |
||||
main.userstat : |
||||
|
||||
# Fails on x86_64 since 10.2.15 |
||||
rocksdb.bulk_load_errors : |
||||
rocksdb.index_merge_rocksdb : |
||||
rocksdb.index_merge_rocksdb2 : |
||||
rocksdb.read_only_tx : |
||||
rocksdb_rpl.mdev12179 : |
||||
rocksdb.shutdown : |
||||
rocksdb.2pc_group_commit : |
||||
|
||||
# Fails on ppc and arm since 10.2.15 |
||||
innodb_gis.rtree_compress2 : |
||||
parts.partition_alter4_innodb : |
||||
|
||||
# Fails from 10.3.9 |
||||
encryption.innodb-redo-badkey : |
||||
|
||||
# Expected: Plugin building disabled in server |
||||
plugins.auth_ed25519 : |
||||
|
||||
# Fails from 10.4.10 |
||||
sys_vars.tcp_nodelay : |
||||
# only on i686 |
||||
main.key_cache : |
||||
parts.optimizer : |
||||
main.opt_trace_index_merge : |
||||
|
@ -0,0 +1,21 @@
@@ -0,0 +1,21 @@
|
||||
# 10.2.12 & 10.2.13 after some uninedtified Rawhide (and the forked f28) buildroot change |
||||
# failed: 1045: Access denied for user '.....'@'localhost' (using password: YES) |
||||
innodb.temporary_table_optimization : |
||||
|
||||
main.derived_cond_pushdown : |
||||
main.ps : |
||||
|
||||
# From 10.3.9 on ppc64le |
||||
encryption.create_or_replace : |
||||
main.select : |
||||
main.select_jcl6 : |
||||
main.select_pkeycache : |
||||
main.sp : |
||||
main.type_float : |
||||
main.type_newdecimal : |
||||
main.type_ranges : |
||||
|
||||
# 10.3.10 |
||||
parts.partition_alter1_2_innodb : |
||||
parts.partition_alter1_1_2_innodb : |
||||
innodb.innodb_defrag_concurrent : |
@ -0,0 +1,9 @@
@@ -0,0 +1,9 @@
|
||||
# Fails since 10.2.15 |
||||
disks.disks : |
||||
|
||||
# Fails since 10.3.9 |
||||
binlog.binlog_flush_binlogs_delete_domain : |
||||
handler.heap : |
||||
handler.interface : |
||||
innodb_fts.innodb_fts_misc : |
||||
rpl.rpl_gtid_delete_domain : |
Loading…
Reference in new issue