Browse Source
* jc/pull-signed-tag: commit-tree: teach -m/-F options to read logs from elsewhere commit-tree: update the command line parsing commit: teach --amend to carry forward extra headers merge: force edit and no-ff mode when merging a tag object commit: copy merged signed tags to headers of merge commit merge: record tag objects without peeling in MERGE_HEAD merge: make usage of commit->util more extensible fmt-merge-msg: Add contents of merged tag in the merge message fmt-merge-msg: package options into a structure fmt-merge-msg: avoid early returns refs DWIMmery: use the same rule for both "git fetch" and others fetch: allow "git fetch $there v1.0" to fetch a tag merge: notice local merging of tags and keep it unwrapped fetch: do not store peeled tag object names in FETCH_HEAD Split GPG interface into its own helper library Conflicts: builtin/fmt-merge-msg.c builtin/merge.cmaint
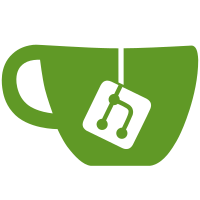
83 changed files with 825 additions and 406 deletions
@ -0,0 +1,138 @@
@@ -0,0 +1,138 @@
|
||||
#include "cache.h" |
||||
#include "run-command.h" |
||||
#include "strbuf.h" |
||||
#include "gpg-interface.h" |
||||
#include "sigchain.h" |
||||
|
||||
static char *configured_signing_key; |
||||
|
||||
void set_signing_key(const char *key) |
||||
{ |
||||
free(configured_signing_key); |
||||
configured_signing_key = xstrdup(key); |
||||
} |
||||
|
||||
int git_gpg_config(const char *var, const char *value, void *cb) |
||||
{ |
||||
if (!strcmp(var, "user.signingkey")) { |
||||
if (!value) |
||||
return config_error_nonbool(var); |
||||
set_signing_key(value); |
||||
} |
||||
return 0; |
||||
} |
||||
|
||||
const char *get_signing_key(void) |
||||
{ |
||||
if (configured_signing_key) |
||||
return configured_signing_key; |
||||
return git_committer_info(IDENT_ERROR_ON_NO_NAME|IDENT_NO_DATE); |
||||
} |
||||
|
||||
/* |
||||
* Create a detached signature for the contents of "buffer" and append |
||||
* it after "signature"; "buffer" and "signature" can be the same |
||||
* strbuf instance, which would cause the detached signature appended |
||||
* at the end. |
||||
*/ |
||||
int sign_buffer(struct strbuf *buffer, struct strbuf *signature, const char *signing_key) |
||||
{ |
||||
struct child_process gpg; |
||||
const char *args[4]; |
||||
ssize_t len; |
||||
size_t i, j, bottom; |
||||
|
||||
memset(&gpg, 0, sizeof(gpg)); |
||||
gpg.argv = args; |
||||
gpg.in = -1; |
||||
gpg.out = -1; |
||||
args[0] = "gpg"; |
||||
args[1] = "-bsau"; |
||||
args[2] = signing_key; |
||||
args[3] = NULL; |
||||
|
||||
if (start_command(&gpg)) |
||||
return error(_("could not run gpg.")); |
||||
|
||||
/* |
||||
* When the username signingkey is bad, program could be terminated |
||||
* because gpg exits without reading and then write gets SIGPIPE. |
||||
*/ |
||||
sigchain_push(SIGPIPE, SIG_IGN); |
||||
|
||||
if (write_in_full(gpg.in, buffer->buf, buffer->len) != buffer->len) { |
||||
close(gpg.in); |
||||
close(gpg.out); |
||||
finish_command(&gpg); |
||||
return error(_("gpg did not accept the data")); |
||||
} |
||||
close(gpg.in); |
||||
|
||||
bottom = signature->len; |
||||
len = strbuf_read(signature, gpg.out, 1024); |
||||
close(gpg.out); |
||||
|
||||
sigchain_pop(SIGPIPE); |
||||
|
||||
if (finish_command(&gpg) || !len || len < 0) |
||||
return error(_("gpg failed to sign the data")); |
||||
|
||||
/* Strip CR from the line endings, in case we are on Windows. */ |
||||
for (i = j = bottom; i < signature->len; i++) |
||||
if (signature->buf[i] != '\r') { |
||||
if (i != j) |
||||
signature->buf[j] = signature->buf[i]; |
||||
j++; |
||||
} |
||||
strbuf_setlen(signature, j); |
||||
|
||||
return 0; |
||||
} |
||||
|
||||
/* |
||||
* Run "gpg" to see if the payload matches the detached signature. |
||||
* gpg_output_to tells where the output from "gpg" should go: |
||||
* < 0: /dev/null |
||||
* = 0: standard error of the calling process |
||||
* > 0: the specified file descriptor |
||||
*/ |
||||
int verify_signed_buffer(const char *payload, size_t payload_size, |
||||
const char *signature, size_t signature_size, |
||||
struct strbuf *gpg_output) |
||||
{ |
||||
struct child_process gpg; |
||||
const char *args_gpg[] = {"gpg", "--verify", "FILE", "-", NULL}; |
||||
char path[PATH_MAX]; |
||||
int fd, ret; |
||||
|
||||
fd = git_mkstemp(path, PATH_MAX, ".git_vtag_tmpXXXXXX"); |
||||
if (fd < 0) |
||||
return error("could not create temporary file '%s': %s", |
||||
path, strerror(errno)); |
||||
if (write_in_full(fd, signature, signature_size) < 0) |
||||
return error("failed writing detached signature to '%s': %s", |
||||
path, strerror(errno)); |
||||
close(fd); |
||||
|
||||
memset(&gpg, 0, sizeof(gpg)); |
||||
gpg.argv = args_gpg; |
||||
gpg.in = -1; |
||||
if (gpg_output) |
||||
gpg.err = -1; |
||||
args_gpg[2] = path; |
||||
if (start_command(&gpg)) { |
||||
unlink(path); |
||||
return error("could not run gpg."); |
||||
} |
||||
|
||||
write_in_full(gpg.in, payload, payload_size); |
||||
close(gpg.in); |
||||
|
||||
if (gpg_output) |
||||
strbuf_read(gpg_output, gpg.err, 0); |
||||
ret = finish_command(&gpg); |
||||
|
||||
unlink_or_warn(path); |
||||
|
||||
return ret; |
||||
} |
@ -0,0 +1,10 @@
@@ -0,0 +1,10 @@
|
||||
#ifndef GPG_INTERFACE_H |
||||
#define GPG_INTERFACE_H |
||||
|
||||
extern int sign_buffer(struct strbuf *buffer, struct strbuf *signature, const char *signing_key); |
||||
extern int verify_signed_buffer(const char *payload, size_t payload_size, const char *signature, size_t signature_size, struct strbuf *gpg_output); |
||||
extern int git_gpg_config(const char *, const char *, void *); |
||||
extern void set_signing_key(const char *); |
||||
extern const char *get_signing_key(void); |
||||
|
||||
#endif |
@ -1,8 +1,8 @@
@@ -1,8 +1,8 @@
|
||||
# br-branches-default |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f branch 'master' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,9 +1,9 @@
@@ -1,9 +1,9 @@
|
||||
# br-branches-default-merge |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge branch 'master' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b branch 'three' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,9 +1,9 @@
@@ -1,9 +1,9 @@
|
||||
# br-branches-default-merge branches-default |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge branch 'master' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b branch 'three' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,8 +1,8 @@
@@ -1,8 +1,8 @@
|
||||
# br-branches-default branches-default |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f branch 'master' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,8 +1,8 @@
@@ -1,8 +1,8 @@
|
||||
# br-branches-one |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 branch 'one' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,9 +1,9 @@
@@ -1,9 +1,9 @@
|
||||
# br-branches-one-merge |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge branch 'one' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b branch 'three' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,9 +1,9 @@
@@ -1,9 +1,9 @@
|
||||
# br-branches-one-merge branches-one |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge branch 'one' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b branch 'three' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,9 +1,9 @@
@@ -1,9 +1,9 @@
|
||||
# br-branches-one-octopus |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 branch 'one' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 branch 'two' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,9 +1,9 @@
@@ -1,9 +1,9 @@
|
||||
# br-branches-one-octopus branches-one |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 branch 'one' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 branch 'two' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,8 +1,8 @@
@@ -1,8 +1,8 @@
|
||||
# br-branches-one branches-one |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 branch 'one' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,7 +1,7 @@
@@ -1,7 +1,7 @@
|
||||
# br-unconfig --tags ../.git |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,7 +1,7 @@
@@ -1,7 +1,7 @@
|
||||
# br-unconfig ../.git tag tag-one-tree tag tag-three-file |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,7 +1,7 @@
@@ -1,7 +1,7 @@
|
||||
# br-unconfig ../.git tag tag-one tag tag-three |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 tag 'tag-one' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b tag 'tag-three' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 tag 'tag-three' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,8 +1,8 @@
@@ -1,8 +1,8 @@
|
||||
# br-unconfig branches-default |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f branch 'master' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,8 +1,8 @@
@@ -1,8 +1,8 @@
|
||||
# br-unconfig branches-one |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 branch 'one' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,7 +1,7 @@
@@ -1,7 +1,7 @@
|
||||
# master --tags ../.git |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,7 +1,7 @@
@@ -1,7 +1,7 @@
|
||||
# master ../.git tag tag-one-tree tag tag-three-file |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,7 +1,7 @@
@@ -1,7 +1,7 @@
|
||||
# master ../.git tag tag-one tag tag-three |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 tag 'tag-one' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b tag 'tag-three' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 tag 'tag-three' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,8 +1,8 @@
@@ -1,8 +1,8 @@
|
||||
# master branches-default |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f branch 'master' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
@ -1,8 +1,8 @@
@@ -1,8 +1,8 @@
|
||||
# master branches-one |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 branch 'one' of ../ |
||||
754b754407bf032e9a2f9d5a9ad05ca79a6b228f not-for-merge tag 'tag-master' of ../ |
||||
6c9dec2b923228c9ff994c6cfe4ae16c12408dc5 not-for-merge tag 'tag-master' of ../ |
||||
8e32a6d901327a23ef831511badce7bf3bf46689 not-for-merge tag 'tag-one' of ../ |
||||
22feea448b023a2d864ef94b013735af34d238ba not-for-merge tag 'tag-one-tree' of ../ |
||||
0567da4d5edd2ff4bb292a465ba9e64dcad9536b not-for-merge tag 'tag-three' of ../ |
||||
c61a82b60967180544e3c19f819ddbd0c9f89899 not-for-merge tag 'tag-three' of ../ |
||||
0e3b14047d3ee365f4f2a1b673db059c3972589c not-for-merge tag 'tag-three-file' of ../ |
||||
6134ee8f857693b96ff1cc98d3e2fd62b199e5a8 not-for-merge tag 'tag-two' of ../ |
||||
525b7fb068d59950d185a8779dc957c77eed73ba not-for-merge tag 'tag-two' of ../ |
||||
|
Loading…
Reference in new issue