Browse Source
This is meant to make raw git not hugely less usable than something like raw CVS. I want to make a 1.0 release of the plumbing, and the actual commit part was just too intimidating.maint
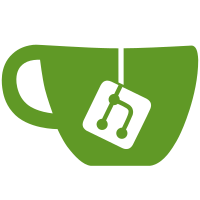
4 changed files with 104 additions and 2 deletions
@ -0,0 +1,15 @@
@@ -0,0 +1,15 @@
|
||||
#!/bin/sh |
||||
git-status-script > .editmsg |
||||
if [ "$?" != "0" ] |
||||
then |
||||
cat .editmsg |
||||
exit 1 |
||||
fi |
||||
ED=${VISUAL:$EDITOR} |
||||
ED=${ED:vi} |
||||
$ED .editmsg |
||||
grep -v '^#' < .editmsg | git-stripspace > .cmitmsg |
||||
[ -s .cmitmsg ] || exit 1 |
||||
tree=$(git-write-tree) || exit 1 |
||||
commit=$(cat .cmitmsg | git-commit-tree $tree -p HEAD) || exit 1 |
||||
echo $commit > .git/HEAD |
@ -0,0 +1,38 @@
@@ -0,0 +1,38 @@
|
||||
#!/bin/sh |
||||
report () { |
||||
header="# |
||||
# $1: |
||||
# ($2) |
||||
# |
||||
" |
||||
trailer="" |
||||
while read oldmode mode oldsha sha status name newname |
||||
do |
||||
echo -n "$header" |
||||
header="" |
||||
trailer="# |
||||
" |
||||
case "$status" in |
||||
M) echo "# modified: $name";; |
||||
D) echo "# deleted: $name";; |
||||
T) echo "# typechange: $name";; |
||||
C) echo "# copied: $name -> $newname";; |
||||
R) echo "# renamed: $name -> $newname";; |
||||
N) echo "# new file: $name";; |
||||
U) echo "# unmerged: $name";; |
||||
esac |
||||
done |
||||
echo -n "$trailer" |
||||
[ "$header" ] |
||||
} |
||||
|
||||
git-update-cache --refresh >& /dev/null |
||||
git-diff-cache -B -C --cached HEAD | sed 's/^://' | report "Updated but not checked in" "will commit" |
||||
committable="$?" |
||||
git-diff-files | sed 's/^://' | report "Changed but not updated" "use git-update-cache to mark for commit" |
||||
if [ "$committable" == "0" ] |
||||
then |
||||
echo "nothing to commit" |
||||
exit 1 |
||||
fi |
||||
exit 0 |
@ -0,0 +1,48 @@
@@ -0,0 +1,48 @@
|
||||
#include <stdio.h> |
||||
#include <string.h> |
||||
#include <ctype.h> |
||||
|
||||
/* |
||||
* Remove empty lines from the beginning and end. |
||||
* |
||||
* Turn multiple consecutive empty lines into just one |
||||
* empty line. |
||||
*/ |
||||
static void cleanup(char *line) |
||||
{ |
||||
int len = strlen(line); |
||||
|
||||
if (len > 1 && line[len-1] == '\n') { |
||||
do { |
||||
unsigned char c = line[len-2]; |
||||
if (!isspace(c)) |
||||
break; |
||||
line[len-2] = '\n'; |
||||
len--; |
||||
line[len] = 0; |
||||
} while (len > 1); |
||||
} |
||||
} |
||||
|
||||
int main(int argc, char **argv) |
||||
{ |
||||
int empties = -1; |
||||
char line[1024]; |
||||
|
||||
while (fgets(line, sizeof(line), stdin)) { |
||||
cleanup(line); |
||||
|
||||
/* Not just an empty line? */ |
||||
if (line[0] != '\n') { |
||||
if (empties > 0) |
||||
putchar('\n'); |
||||
empties = 0; |
||||
fputs(line, stdout); |
||||
continue; |
||||
} |
||||
if (empties < 0) |
||||
continue; |
||||
empties++; |
||||
} |
||||
return 0; |
||||
} |
Loading…
Reference in new issue