Browse Source
A few subcommands have been taught to stop users from working on a branch that is being used in another worktree linked to the same repository. * rj/avoid-switching-to-already-used-branch: switch: reject if the branch is already checked out elsewhere (test) rebase: refuse to switch to a branch already checked out elsewhere (test) branch: fix die_if_checked_out() when ignore_current_worktree worktree: introduce is_shared_symref()main
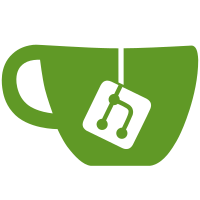
5 changed files with 89 additions and 37 deletions
Loading…
Reference in new issue