Browse Source
* commit '633e3556ccbc': (5835 commits) build-in git-mktree allow -t abbreviation for --track in git branch gitweb: Remove function prototypes (cleanup) Documentation: cloning to empty directory is allowed Clarify kind of conflict in merge-one-file helper git config: clarify --add and --get-color archive-tar.c: squelch a type mismatch warning Start 1.6.4 development Start 1.6.3.1 maintenance series. GIT 1.6.3 t4029: use sh instead of bash t4200: convert sed expression which operates on non-text file to perl t4200: remove two unnecessary lines t/annotate-tests.sh: avoid passing a non-newline terminated file to sed t4118: avoid sed invocation on file without terminating newline t4118: add missing '&&' t8005: use egrep when extended regular expressions are required git-clean doc: the command only affects paths under $(cwd) improve error message in config.c t4018-diff-funcname: add cpp xfuncname pattern to syntax test ...maint
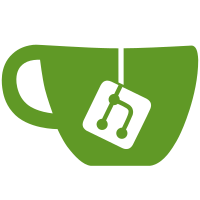
1366 changed files with 172957 additions and 45557 deletions
@ -0,0 +1,2 @@
@@ -0,0 +1,2 @@
|
||||
* whitespace=!indent,trail,space |
||||
*.[ch] whitespace |
@ -0,0 +1 @@
@@ -0,0 +1 @@
|
||||
*.txt whitespace |
@ -0,0 +1,134 @@
@@ -0,0 +1,134 @@
|
||||
Like other projects, we also have some guidelines to keep to the |
||||
code. For git in general, three rough rules are: |
||||
|
||||
- Most importantly, we never say "It's in POSIX; we'll happily |
||||
ignore your needs should your system not conform to it." |
||||
We live in the real world. |
||||
|
||||
- However, we often say "Let's stay away from that construct, |
||||
it's not even in POSIX". |
||||
|
||||
- In spite of the above two rules, we sometimes say "Although |
||||
this is not in POSIX, it (is so convenient | makes the code |
||||
much more readable | has other good characteristics) and |
||||
practically all the platforms we care about support it, so |
||||
let's use it". |
||||
|
||||
Again, we live in the real world, and it is sometimes a |
||||
judgement call, the decision based more on real world |
||||
constraints people face than what the paper standard says. |
||||
|
||||
|
||||
As for more concrete guidelines, just imitate the existing code |
||||
(this is a good guideline, no matter which project you are |
||||
contributing to). It is always preferable to match the _local_ |
||||
convention. New code added to git suite is expected to match |
||||
the overall style of existing code. Modifications to existing |
||||
code is expected to match the style the surrounding code already |
||||
uses (even if it doesn't match the overall style of existing code). |
||||
|
||||
But if you must have a list of rules, here they are. |
||||
|
||||
For shell scripts specifically (not exhaustive): |
||||
|
||||
- We prefer $( ... ) for command substitution; unlike ``, it |
||||
properly nests. It should have been the way Bourne spelled |
||||
it from day one, but unfortunately isn't. |
||||
|
||||
- We use ${parameter-word} and its [-=?+] siblings, and their |
||||
colon'ed "unset or null" form. |
||||
|
||||
- We use ${parameter#word} and its [#%] siblings, and their |
||||
doubled "longest matching" form. |
||||
|
||||
- We use Arithmetic Expansion $(( ... )). |
||||
|
||||
- No "Substring Expansion" ${parameter:offset:length}. |
||||
|
||||
- No shell arrays. |
||||
|
||||
- No strlen ${#parameter}. |
||||
|
||||
- No regexp ${parameter/pattern/string}. |
||||
|
||||
- We do not use Process Substitution <(list) or >(list). |
||||
|
||||
- We prefer "test" over "[ ... ]". |
||||
|
||||
- We do not write the noiseword "function" in front of shell |
||||
functions. |
||||
|
||||
- As to use of grep, stick to a subset of BRE (namely, no \{m,n\}, |
||||
[::], [==], nor [..]) for portability. |
||||
|
||||
- We do not use \{m,n\}; |
||||
|
||||
- We do not use -E; |
||||
|
||||
- We do not use ? nor + (which are \{0,1\} and \{1,\} |
||||
respectively in BRE) but that goes without saying as these |
||||
are ERE elements not BRE (note that \? and \+ are not even part |
||||
of BRE -- making them accessible from BRE is a GNU extension). |
||||
|
||||
For C programs: |
||||
|
||||
- We use tabs to indent, and interpret tabs as taking up to |
||||
8 spaces. |
||||
|
||||
- We try to keep to at most 80 characters per line. |
||||
|
||||
- When declaring pointers, the star sides with the variable |
||||
name, i.e. "char *string", not "char* string" or |
||||
"char * string". This makes it easier to understand code |
||||
like "char *string, c;". |
||||
|
||||
- We avoid using braces unnecessarily. I.e. |
||||
|
||||
if (bla) { |
||||
x = 1; |
||||
} |
||||
|
||||
is frowned upon. A gray area is when the statement extends |
||||
over a few lines, and/or you have a lengthy comment atop of |
||||
it. Also, like in the Linux kernel, if there is a long list |
||||
of "else if" statements, it can make sense to add braces to |
||||
single line blocks. |
||||
|
||||
- We try to avoid assignments inside if(). |
||||
|
||||
- Try to make your code understandable. You may put comments |
||||
in, but comments invariably tend to stale out when the code |
||||
they were describing changes. Often splitting a function |
||||
into two makes the intention of the code much clearer. |
||||
|
||||
- Double negation is often harder to understand than no negation |
||||
at all. |
||||
|
||||
- Some clever tricks, like using the !! operator with arithmetic |
||||
constructs, can be extremely confusing to others. Avoid them, |
||||
unless there is a compelling reason to use them. |
||||
|
||||
- Use the API. No, really. We have a strbuf (variable length |
||||
string), several arrays with the ALLOC_GROW() macro, a |
||||
string_list for sorted string lists, a hash map (mapping struct |
||||
objects) named "struct decorate", amongst other things. |
||||
|
||||
- When you come up with an API, document it. |
||||
|
||||
- The first #include in C files, except in platform specific |
||||
compat/ implementations, should be git-compat-util.h or another |
||||
header file that includes it, such as cache.h or builtin.h. |
||||
|
||||
- If you are planning a new command, consider writing it in shell |
||||
or perl first, so that changes in semantics can be easily |
||||
changed and discussed. Many git commands started out like |
||||
that, and a few are still scripts. |
||||
|
||||
- Avoid introducing a new dependency into git. This means you |
||||
usually should stay away from scripting languages not already |
||||
used in the git core command set (unless your command is clearly |
||||
separate from it, such as an importer to convert random-scm-X |
||||
repositories to git). |
||||
|
||||
- When we pass <string, length> pair to functions, we should try to |
||||
pass them in that order. |
@ -0,0 +1,58 @@
@@ -0,0 +1,58 @@
|
||||
GIT v1.5.3.2 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.3.1 |
||||
-------------------- |
||||
|
||||
* git-push sent thin packs by default, which was not good for |
||||
the public distribution server (no point in saving transfer |
||||
while pushing; no point in making the resulting pack less |
||||
optimum). |
||||
|
||||
* git-svn sometimes terminated with "Malformed network data" when |
||||
talking over svn:// protocol. |
||||
|
||||
* git-send-email re-issued the same message-id about 10% of the |
||||
time if you fired off 30 messages within a single second. |
||||
|
||||
* git-stash was not terminating the log message of commits it |
||||
internally creates with LF. |
||||
|
||||
* git-apply failed to check the size of the patch hunk when its |
||||
beginning part matched the remainder of the preimage exactly, |
||||
even though the preimage recorded in the hunk was much larger |
||||
(therefore the patch should not have applied), leading to a |
||||
segfault. |
||||
|
||||
* "git rm foo && git commit foo" complained that 'foo' needs to |
||||
be added first, instead of committing the removal, which was a |
||||
nonsense. |
||||
|
||||
* git grep -c said "/dev/null: 0". |
||||
|
||||
* git-add -u failed to recognize a blob whose type changed |
||||
between the index and the work tree. |
||||
|
||||
* The limit to rename detection has been tightened a lot to |
||||
reduce performance problems with a huge change. |
||||
|
||||
* cvsimport and svnimport barfed when the input tried to move |
||||
a tag. |
||||
|
||||
* "git apply -pN" did not chop the right number of directories. |
||||
|
||||
* "git svnimport" did not like SVN tags with funny characters in them. |
||||
|
||||
* git-gui 0.8.3, with assorted fixes, including: |
||||
|
||||
- font-chooser on X11 was unusable with large number of fonts; |
||||
- a diff that contained a deleted symlink made it barf; |
||||
- an untracked symbolic link to a directory made it fart; |
||||
- a file with % in its name made it vomit; |
||||
|
||||
|
||||
Documentation updates |
||||
--------------------- |
||||
|
||||
User manual has been somewhat restructured. I think the new |
||||
organization is much easier to read. |
@ -0,0 +1,31 @@
@@ -0,0 +1,31 @@
|
||||
GIT v1.5.3.3 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.3.2 |
||||
-------------------- |
||||
|
||||
* git-quiltimport did not like it when a patch described in the |
||||
series file does not exist. |
||||
|
||||
* p4 importer missed executable bit in some cases. |
||||
|
||||
* The default shell on some FreeBSD did not execute the |
||||
argument parsing code correctly and made git unusable. |
||||
|
||||
* git-svn incorrectly spawned pager even when the user |
||||
explicitly asked not to. |
||||
|
||||
* sample post-receive hook overquoted the envelope sender |
||||
value. |
||||
|
||||
* git-am got confused when the patch contained a change that is |
||||
only about type and not contents. |
||||
|
||||
* git-mergetool did not show our and their version of the |
||||
conflicted file when started from a subdirectory of the |
||||
project. |
||||
|
||||
* git-mergetool did not pass correct options when invoking diff3. |
||||
|
||||
* git-log sometimes invoked underlying "diff" machinery |
||||
unnecessarily. |
@ -0,0 +1,35 @@
@@ -0,0 +1,35 @@
|
||||
GIT v1.5.3.4 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.3.3 |
||||
-------------------- |
||||
|
||||
* Change to "git-ls-files" in v1.5.3.3 that was introduced to support |
||||
partial commit of removal better had a segfaulting bug, which was |
||||
diagnosed and fixed by Keith and Carl. |
||||
|
||||
* Performance improvements for rename detection has been backported |
||||
from the 'master' branch. |
||||
|
||||
* "git-for-each-ref --format='%(numparent)'" was not working |
||||
correctly at all, and --format='%(parent)' was not working for |
||||
merge commits. |
||||
|
||||
* Sample "post-receive-hook" incorrectly sent out push |
||||
notification e-mails marked as "From: " the committer of the |
||||
commit that happened to be at the tip of the branch that was |
||||
pushed, not from the person who pushed. |
||||
|
||||
* "git-remote" did not exit non-zero status upon error. |
||||
|
||||
* "git-add -i" did not respond very well to EOF from tty nor |
||||
bogus input. |
||||
|
||||
* "git-rebase -i" squash subcommand incorrectly made the |
||||
author of later commit the author of resulting commit, |
||||
instead of taking from the first one in the squashed series. |
||||
|
||||
* "git-stash apply --index" was not documented. |
||||
|
||||
* autoconfiguration learned that "ar" command is found as "gas" on |
||||
some systems. |
@ -0,0 +1,94 @@
@@ -0,0 +1,94 @@
|
||||
GIT v1.5.3.5 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.3.4 |
||||
-------------------- |
||||
|
||||
* Comes with git-gui 0.8.4. |
||||
|
||||
* "git-config" silently ignored options after --list; now it will |
||||
error out with a usage message. |
||||
|
||||
* "git-config --file" failed if the argument used a relative path |
||||
as it changed directories before opening the file. |
||||
|
||||
* "git-config --file" now displays a proper error message if it |
||||
cannot read the file specified on the command line. |
||||
|
||||
* "git-config", "git-diff", "git-apply" failed if run from a |
||||
subdirectory with relative GIT_DIR and GIT_WORK_TREE set. |
||||
|
||||
* "git-blame" crashed if run during a merge conflict. |
||||
|
||||
* "git-add -i" did not handle single line hunks correctly. |
||||
|
||||
* "git-rebase -i" and "git-stash apply" failed if external diff |
||||
drivers were used for one or more files in a commit. They now |
||||
avoid calling the external diff drivers. |
||||
|
||||
* "git-log --follow" did not work unless diff generation (e.g. -p) |
||||
was also requested. |
||||
|
||||
* "git-log --follow -B" did not work at all. Fixed. |
||||
|
||||
* "git-log -M -B" did not correctly handle cases of very large files |
||||
being renamed and replaced by very small files in the same commit. |
||||
|
||||
* "git-log" printed extra newlines between commits when a diff |
||||
was generated internally (e.g. -S or --follow) but not displayed. |
||||
|
||||
* "git-push" error message is more helpful when pushing to a |
||||
repository with no matching refs and none specified. |
||||
|
||||
* "git-push" now respects + (force push) on wildcard refspecs, |
||||
matching the behavior of git-fetch. |
||||
|
||||
* "git-filter-branch" now updates the working directory when it |
||||
has finished filtering the current branch. |
||||
|
||||
* "git-instaweb" no longer fails on Mac OS X. |
||||
|
||||
* "git-cvsexportcommit" didn't always create new parent directories |
||||
before trying to create new child directories. Fixed. |
||||
|
||||
* "git-fetch" printed a scary (but bogus) error message while |
||||
fetching a tag that pointed to a tree or blob. The error did |
||||
not impact correctness, only user perception. The bogus error |
||||
is no longer printed. |
||||
|
||||
* "git-ls-files --ignored" did not properly descend into non-ignored |
||||
directories that themselves contained ignored files if d_type |
||||
was not supported by the filesystem. This bug impacted systems |
||||
such as AFS. Fixed. |
||||
|
||||
* Git segfaulted when reading an invalid .gitattributes file. Fixed. |
||||
|
||||
* post-receive-email example hook was fixed for non-fast-forward |
||||
updates. |
||||
|
||||
* Documentation updates for supported (but previously undocumented) |
||||
options of "git-archive" and "git-reflog". |
||||
|
||||
* "make clean" no longer deletes the configure script that ships |
||||
with the git tarball, making multiple architecture builds easier. |
||||
|
||||
* "git-remote show origin" spewed a warning message from Perl |
||||
when no remote is defined for the current branch via |
||||
branch.<name>.remote configuration settings. |
||||
|
||||
* Building with NO_PERL_MAKEMAKER excessively rebuilt contents |
||||
of perl/ subdirectory by rewriting perl.mak. |
||||
|
||||
* http.sslVerify configuration settings were not used in scripted |
||||
Porcelains. |
||||
|
||||
* "git-add" leaked a bit of memory while scanning for files to add. |
||||
|
||||
* A few workarounds to squelch false warnings from recent gcc have |
||||
been added. |
||||
|
||||
* "git-send-pack $remote frotz" segfaulted when there is nothing |
||||
named 'frotz' on the local end. |
||||
|
||||
* "git-rebase --interactive" did not handle its "--strategy" option |
||||
properly. |
@ -0,0 +1,48 @@
@@ -0,0 +1,48 @@
|
||||
GIT v1.5.3.6 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.3.5 |
||||
-------------------- |
||||
|
||||
* git-cvsexportcommit handles root commits better. |
||||
|
||||
* git-svn dcommit used to clobber when sending a series of |
||||
patches. |
||||
|
||||
* git-svn dcommit failed after attempting to rebase when |
||||
started with a dirty index; now it stops upfront. |
||||
|
||||
* git-grep sometimes refused to work when your index was |
||||
unmerged. |
||||
|
||||
* "git-grep -A1 -B2" acted as if it was told to run "git -A1 -B21". |
||||
|
||||
* git-hash-object did not honor configuration variables, such as |
||||
core.compression. |
||||
|
||||
* git-index-pack choked on a huge pack on 32-bit machines, even when |
||||
large file offsets are supported. |
||||
|
||||
* atom feeds from git-web said "10" for the month of November. |
||||
|
||||
* a memory leak in commit walker was plugged. |
||||
|
||||
* When git-send-email inserted the original author's From: |
||||
address in body, it did not mark the message with |
||||
Content-type: as needed. |
||||
|
||||
* git-revert and git-cherry-pick incorrectly refused to start |
||||
when the work tree was dirty. |
||||
|
||||
* git-clean did not honor core.excludesfile configuration. |
||||
|
||||
* git-add mishandled ".gitignore" files when applying them to |
||||
subdirectories. |
||||
|
||||
* While importing a too branchy history, git-fastimport did not |
||||
honor delta depth limit properly. |
||||
|
||||
* Support for zlib implementations that lack ZLIB_VERNUM and definition |
||||
of deflateBound() has been added. |
||||
|
||||
* Quite a lot of documentation clarifications. |
@ -0,0 +1,45 @@
@@ -0,0 +1,45 @@
|
||||
GIT v1.5.3.7 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.3.6 |
||||
-------------------- |
||||
|
||||
* git-send-email added 8-bit contents to the payload without |
||||
marking it as 8-bit in a CTE header. |
||||
|
||||
* "git-bundle create a.bndl HEAD" dereferenced the symref and |
||||
did not record the ref as 'HEAD'; this prevented a bundle |
||||
from being used as a normal source of git-clone. |
||||
|
||||
* The code to reject nonsense command line of the form |
||||
"git-commit -a paths..." and "git-commit --interactive |
||||
paths..." were broken. |
||||
|
||||
* Adding a signature that is not ASCII-only to an original |
||||
commit that is ASCII-only would make the result non-ASCII. |
||||
"git-format-patch -s" did not mark such a message correctly |
||||
with MIME encoding header. |
||||
|
||||
* git-add sometimes did not mark the resulting index entry |
||||
stat-clean. This affected only cases when adding the |
||||
contents with the same length as the previously staged |
||||
contents, and the previous staging made the index entry |
||||
"racily clean". |
||||
|
||||
* git-commit did not honor GIT_INDEX_FILE the user had in the |
||||
environment. |
||||
|
||||
* When checking out a revision, git-checkout did not report where the |
||||
updated HEAD is if you happened to have a file called HEAD in the |
||||
work tree. |
||||
|
||||
* "git-rev-list --objects" mishandled a tree that points at a |
||||
submodule. |
||||
|
||||
* "git cvsimport" was not ready for packed refs that "git gc" can |
||||
produce and gave incorrect results. |
||||
|
||||
* Many scripted Porcelains were confused when you happened to have a |
||||
file called "HEAD" in your work tree. |
||||
|
||||
Also it contains updates to the user manual and documentation. |
@ -0,0 +1,25 @@
@@ -0,0 +1,25 @@
|
||||
GIT v1.5.3.8 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.3.7 |
||||
-------------------- |
||||
|
||||
* Some documentation used "email.com" as an example domain. |
||||
|
||||
* git-svn fix to handle funky branch and project names going over |
||||
http/https correctly. |
||||
|
||||
* git-svn fix to tone down a needlessly alarming warning message. |
||||
|
||||
* git-clone did not correctly report errors while fetching over http. |
||||
|
||||
* git-send-email added redundant Message-Id: header to the outgoing |
||||
e-mail when the patch text already had one. |
||||
|
||||
* a read-beyond-end-of-buffer bug in configuration file updater was fixed. |
||||
|
||||
* git-grep used to show the same hit repeatedly for unmerged paths. |
||||
|
||||
* After amending the patch title in "git-am -i", the command did not |
||||
report the patch it applied with the updated title. |
||||
|
@ -0,0 +1,17 @@
@@ -0,0 +1,17 @@
|
||||
GIT v1.5.4.1 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.4 |
||||
------------------ |
||||
|
||||
* "git-commit -C $tag" used to work but rewrite in C done in |
||||
1.5.4 broke it. |
||||
|
||||
* An entry in the .gitattributes file that names a pattern in a |
||||
subdirectory of the directory it is in did not match |
||||
correctly (e.g. pattern "b/*.c" in "a/.gitattributes" should |
||||
match "a/b/foo.c" but it didn't). |
||||
|
||||
* Customized color specification was parsed incorrectly when |
||||
numeric color values are used. This was fixed in 1.5.4.1. |
||||
|
@ -0,0 +1,43 @@
@@ -0,0 +1,43 @@
|
||||
GIT v1.5.4.2 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.4 |
||||
------------------ |
||||
|
||||
* The configuration parser was not prepared to see string |
||||
valued variables misspelled as boolean and segfaulted. |
||||
|
||||
* Temporary files left behind due to interrupted object |
||||
transfers were not cleaned up with "git prune". |
||||
|
||||
* "git config --unset" was confused when the unset variables |
||||
were spelled with continuation lines in the config file. |
||||
|
||||
* The merge message detection in "git cvsimport" did not catch |
||||
a message that began with "Merge...". |
||||
|
||||
* "git status" suggests "git rm --cached" for unstaging the |
||||
earlier "git add" before the initial commit. |
||||
|
||||
* "git status" output was incorrect during a partial commit. |
||||
|
||||
* "git bisect" refused to start when the HEAD was detached. |
||||
|
||||
* "git bisect" allowed a wildcard character in the commit |
||||
message expanded while writing its log file. |
||||
|
||||
* Manual pages were not formatted correctly with docbook xsl |
||||
1.72; added a workaround. |
||||
|
||||
* "git-commit -C $tag" used to work but rewrite in C done in |
||||
1.5.4 broke it. This was fixed in 1.5.4.1. |
||||
|
||||
* An entry in the .gitattributes file that names a pattern in a |
||||
subdirectory of the directory it is in did not match |
||||
correctly (e.g. pattern "b/*.c" in "a/.gitattributes" should |
||||
match "a/b/foo.c" but it didn't). This was fixed in 1.5.4.1. |
||||
|
||||
* Customized color specification was parsed incorrectly when |
||||
numeric color values are used. This was fixed in 1.5.4.1. |
||||
|
||||
* http transport misbehaved when linked with curl-gnutls. |
@ -0,0 +1,27 @@
@@ -0,0 +1,27 @@
|
||||
GIT v1.5.4.3 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.4.2 |
||||
-------------------- |
||||
|
||||
* RPM spec used to pull in everything with 'git'. This has been |
||||
changed so that 'git' package contains just the core parts, |
||||
and we now supply 'git-all' metapackage to slurp in everything. |
||||
This should match end user's expectation better. |
||||
|
||||
* When some refs failed to update, git-push reported "failure" |
||||
which was unclear if some other refs were updated or all of |
||||
them failed atomically (the answer is the former). Reworded |
||||
the message to clarify this. |
||||
|
||||
* "git clone" from a repository whose HEAD was misconfigured |
||||
did not set up the remote properly. Now it tries to do |
||||
better. |
||||
|
||||
* Updated git-push documentation to clarify what "matching" |
||||
means, in order to reduce user confusion. |
||||
|
||||
* Updated git-add documentation to clarify "add -u" operates in |
||||
the current subdirectory you are in, just like other commands. |
||||
|
||||
* git-gui updates to work on OSX and Windows better. |
@ -0,0 +1,66 @@
@@ -0,0 +1,66 @@
|
||||
GIT v1.5.4.4 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.4.3 |
||||
-------------------- |
||||
|
||||
* Building and installing with an overtight umask such as 077 made |
||||
installed templates unreadable by others, while the rest of the install |
||||
are done in a way that is friendly to umask 022. |
||||
|
||||
* "git cvsexportcommit -w $cvsdir" misbehaved when GIT_DIR is set to a |
||||
relative directory. |
||||
|
||||
* "git http-push" had an invalid memory access that could lead it to |
||||
segfault. |
||||
|
||||
* When "git rebase -i" gave control back to the user for a commit that is |
||||
marked to be edited, it just said "modify it with commit --amend", |
||||
without saying what to do to continue after modifying it. Give an |
||||
explicit instruction to run "rebase --continue" to be more helpful. |
||||
|
||||
* "git send-email" in 1.5.4.3 issued a bogus empty In-Reply-To: header. |
||||
|
||||
* "git bisect" showed mysterious "won't bisect on seeked tree" error message. |
||||
This was leftover from Cogito days to prevent "bisect" starting from a |
||||
cg-seeked state. We still keep the Cogito safety, but running "git bisect |
||||
start" when another bisect was in effect will clean up and start over. |
||||
|
||||
* "git push" with an explicit PATH to receive-pack did not quite work if |
||||
receive-pack was not on usual PATH. We earlier fixed the same issue |
||||
with "git fetch" and upload-pack, but somehow forgot to do so in the |
||||
other direction. |
||||
|
||||
* git-gui's info dialog was not displayed correctly when the user tries |
||||
to commit nothing (i.e. without staging anything). |
||||
|
||||
* "git revert" did not properly fail when attempting to run with a |
||||
dirty index. |
||||
|
||||
* "git merge --no-commit --no-ff <other>" incorrectly made commits. |
||||
|
||||
* "git merge --squash --no-ff <other>", which is a nonsense combination |
||||
of options, was not rejected. |
||||
|
||||
* "git ls-remote" and "git remote show" against an empty repository |
||||
failed, instead of just giving an empty result (regression). |
||||
|
||||
* "git fast-import" did not handle a renamed path whose name needs to be |
||||
quoted, due to a bug in unquote_c_style() function. |
||||
|
||||
* "git cvsexportcommit" was confused when multiple files with the same |
||||
basename needed to be pushed out in the same commit. |
||||
|
||||
* "git daemon" did not send early errors to syslog. |
||||
|
||||
* "git log --merge" did not work well with --left-right option. |
||||
|
||||
* "git svn" prompted for client cert password every time it accessed the |
||||
server. |
||||
|
||||
* The reset command in "git fast-import" data stream was documented to |
||||
end with an optional LF, but it actually required one. |
||||
|
||||
* "git svn dcommit/rebase" did not honor --rewrite-root option. |
||||
|
||||
Also included are a handful documentation updates. |
@ -0,0 +1,56 @@
@@ -0,0 +1,56 @@
|
||||
GIT v1.5.4.5 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.4.4 |
||||
-------------------- |
||||
|
||||
* "git fetch there" when the URL information came from the Cogito style |
||||
branches/there file did not update refs/heads/there (regression in |
||||
1.5.4). |
||||
|
||||
* Bogus refspec configuration such as "remote.there.fetch = =" were not |
||||
detected as errors (regression in 1.5.4). |
||||
|
||||
* You couldn't specify a custom editor whose path contains a whitespace |
||||
via GIT_EDITOR (and core.editor). |
||||
|
||||
* The subdirectory filter to "git filter-branch" mishandled a history |
||||
where the subdirectory becomes empty and then later becomes non-empty. |
||||
|
||||
* "git shortlog" gave an empty line if the original commit message was |
||||
malformed (e.g. a botched import from foreign SCM). Now it finds the |
||||
first non-empty line and uses it for better information. |
||||
|
||||
* When the user fails to give a revision parameter to "git svn", an error |
||||
from the Perl interpreter was issued because the script lacked proper |
||||
error checking. |
||||
|
||||
* After "git rebase" stopped due to conflicts, if the user played with |
||||
"git reset" and friends, "git rebase --abort" failed to go back to the |
||||
correct commit. |
||||
|
||||
* Additional work trees prepared with git-new-workdir (in contrib/) did |
||||
not share git-svn metadata directory .git/svn with the original. |
||||
|
||||
* "git-merge-recursive" did not mark addition of the same path with |
||||
different filemodes correctly as a conflict. |
||||
|
||||
* "gitweb" gave malformed URL when pathinfo stype paths are in use. |
||||
|
||||
* "-n" stands for "--no-tags" again for "git fetch". |
||||
|
||||
* "git format-patch" did not detect the need to add 8-bit MIME header |
||||
when the user used format.header configuration. |
||||
|
||||
* "rev~" revision specifier used to mean "rev", which was inconsistent |
||||
with how "rev^" worked. Now "rev~" is the same as "rev~1" (hence it |
||||
also is the same as "rev^1"), and "rev~0" is the same as "rev^0" |
||||
(i.e. it has to be a commit). |
||||
|
||||
* "git quiltimport" did not grok empty lines, lines in "file -pNNN" |
||||
format to specify the prefix levels and lines with trailing comments. |
||||
|
||||
* "git rebase -m" triggered pre-commit verification, which made |
||||
"rebase --continue" impossible. |
||||
|
||||
As usual, it also comes with many documentation fixes and clarifications. |
@ -0,0 +1,43 @@
@@ -0,0 +1,43 @@
|
||||
GIT v1.5.4.6 Release Notes |
||||
========================== |
||||
|
||||
I personally do not think there is any reason anybody should want to |
||||
run v1.5.4.X series these days, because 'master' version is always |
||||
more stable than any tagged released version of git. |
||||
|
||||
This is primarily to futureproof "git-shell" to accept requests |
||||
without a dash between "git" and subcommand name (e.g. "git |
||||
upload-pack") which the newer client will start to make sometime in |
||||
the future. |
||||
|
||||
Fixes since v1.5.4.5 |
||||
-------------------- |
||||
|
||||
* Command line option "-n" to "git-repack" was not correctly parsed. |
||||
|
||||
* Error messages from "git-apply" when the patchfile cannot be opened |
||||
have been improved. |
||||
|
||||
* Error messages from "git-bisect" when given nonsense revisions have |
||||
been improved. |
||||
|
||||
* reflog syntax that uses time e.g. "HEAD@{10 seconds ago}:path" did not |
||||
stop parsing at the closing "}". |
||||
|
||||
* "git rev-parse --symbolic-full-name ^master^2" printed solitary "^", |
||||
but it should print nothing. |
||||
|
||||
* "git apply" did not enforce "match at the beginning" correctly. |
||||
|
||||
* a path specification "a/b" in .gitattributes file should not match |
||||
"sub/a/b", but it did. |
||||
|
||||
* "git log --date-order --topo-order" did not override the earlier |
||||
date-order with topo-order as expected. |
||||
|
||||
* "git fast-export" did not export octopus merges correctly. |
||||
|
||||
* "git archive --prefix=$path/" mishandled gitattributes. |
||||
|
||||
As usual, it also comes with many documentation fixes and clarifications. |
||||
|
@ -0,0 +1,10 @@
@@ -0,0 +1,10 @@
|
||||
GIT v1.5.4.7 Release Notes |
||||
========================== |
||||
|
||||
Fixes since 1.5.4.7 |
||||
------------------- |
||||
|
||||
* Removed support for an obsolete gitweb request URI, whose |
||||
implementation ran "git diff" Porcelain, instead of using plumbing, |
||||
which would have run an external diff command specified in the |
||||
repository configuration as the gitweb user. |
@ -0,0 +1,44 @@
@@ -0,0 +1,44 @@
|
||||
GIT v1.5.5.1 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.5 |
||||
------------------ |
||||
|
||||
* "git archive --prefix=$path/" mishandled gitattributes. |
||||
|
||||
* "git fetch -v" that fetches into FETCH_HEAD did not report the summary |
||||
the same way as done for updating the tracking refs. |
||||
|
||||
* "git svn" misbehaved when the configuration file customized the "git |
||||
log" output format using format.pretty. |
||||
|
||||
* "git submodule status" leaked an unnecessary error message. |
||||
|
||||
* "git log --date-order --topo-order" did not override the earlier |
||||
date-order with topo-order as expected. |
||||
|
||||
* "git bisect good $this" did not check the validity of the revision |
||||
given properly. |
||||
|
||||
* "url.<there>.insteadOf" did not work correctly. |
||||
|
||||
* "git clean" ran inside subdirectory behaved as if the directory was |
||||
explicitly specified for removal by the end user from the top level. |
||||
|
||||
* "git bisect" from a detached head leaked an unnecessary error message. |
||||
|
||||
* "git bisect good $a $b" when $a is Ok but $b is bogus should have |
||||
atomically failed before marking $a as good. |
||||
|
||||
* "git fmt-merge-msg" did not clean up leading empty lines from commit |
||||
log messages like "git log" family does. |
||||
|
||||
* "git am" recorded a commit with empty Subject: line without |
||||
complaining. |
||||
|
||||
* when given a commit log message whose first paragraph consists of |
||||
multiple lines, "git rebase" squashed it into a single line. |
||||
|
||||
* "git remote add $bogus_name $url" did not complain properly. |
||||
|
||||
Also comes with various documentation updates. |
@ -0,0 +1,27 @@
@@ -0,0 +1,27 @@
|
||||
GIT v1.5.5.2 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.5.1 |
||||
-------------------- |
||||
|
||||
* "git repack -n" was mistakenly made no-op earlier. |
||||
|
||||
* "git imap-send" wanted to always have imap.host even when use of |
||||
imap.tunnel made it unnecessary. |
||||
|
||||
* reflog syntax that uses time e.g. "HEAD@{10 seconds ago}:path" did not |
||||
stop parsing at the closing "}". |
||||
|
||||
* "git rev-parse --symbolic-full-name ^master^2" printed solitary "^", |
||||
but it should print nothing. |
||||
|
||||
* "git commit" did not detect when it failed to write tree objects. |
||||
|
||||
* "git fetch" sometimes transferred too many objects unnecessarily. |
||||
|
||||
* a path specification "a/b" in .gitattributes file should not match |
||||
"sub/a/b". |
||||
|
||||
* various gitweb fixes. |
||||
|
||||
Also comes with various documentation updates. |
@ -0,0 +1,12 @@
@@ -0,0 +1,12 @@
|
||||
GIT v1.5.5.3 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.5.2 |
||||
-------------------- |
||||
|
||||
* "git send-email --compose" did not notice that non-ascii contents |
||||
needed some MIME magic. |
||||
|
||||
* "git fast-export" did not export octopus merges correctly. |
||||
|
||||
Also comes with various documentation updates. |
@ -0,0 +1,7 @@
@@ -0,0 +1,7 @@
|
||||
GIT v1.5.5.4 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.5.4 |
||||
-------------------- |
||||
|
||||
* "git name-rev --all" used to segfault. |
@ -0,0 +1,11 @@
@@ -0,0 +1,11 @@
|
||||
GIT v1.5.5.5 Release Notes |
||||
========================== |
||||
|
||||
I personally do not think there is any reason anybody should want to |
||||
run v1.5.5.X series these days, because 'master' version is always |
||||
more stable than any tagged released version of git. |
||||
|
||||
This is primarily to futureproof "git-shell" to accept requests |
||||
without a dash between "git" and subcommand name (e.g. "git |
||||
upload-pack") which the newer client will start to make sometime in |
||||
the future. |
@ -0,0 +1,10 @@
@@ -0,0 +1,10 @@
|
||||
GIT v1.5.5.6 Release Notes |
||||
========================== |
||||
|
||||
Fixes since 1.5.5.5 |
||||
------------------- |
||||
|
||||
* Removed support for an obsolete gitweb request URI, whose |
||||
implementation ran "git diff" Porcelain, instead of using plumbing, |
||||
which would have run an external diff command specified in the |
||||
repository configuration as the gitweb user. |
@ -0,0 +1,207 @@
@@ -0,0 +1,207 @@
|
||||
GIT v1.5.5 Release Notes |
||||
======================== |
||||
|
||||
Updates since v1.5.4 |
||||
-------------------- |
||||
|
||||
(subsystems) |
||||
|
||||
* Comes with git-gui 0.10.1 |
||||
|
||||
(portability) |
||||
|
||||
* We shouldn't ask for BSD group ownership semantics by setting g+s bit |
||||
on directories on older BSD systems that refuses chmod() by non root |
||||
users. BSD semantics is the default there anyway. |
||||
|
||||
* Bunch of portability improvement patches coming from an effort to port |
||||
to Solaris has been applied. |
||||
|
||||
(performance) |
||||
|
||||
* On platforms with suboptimal qsort(3) implementation, there |
||||
is an option to use more reasonable substitute we ship with |
||||
our software. |
||||
|
||||
* New configuration variable "pack.packsizelimit" can be used |
||||
in place of command line option --max-pack-size. |
||||
|
||||
* "git fetch" over the native git protocol used to make a |
||||
connection to find out the set of current remote refs and |
||||
another to actually download the pack data. We now use only |
||||
one connection for these tasks. |
||||
|
||||
* "git commit" does not run lstat(2) more than necessary |
||||
anymore. |
||||
|
||||
(usability, bells and whistles) |
||||
|
||||
* Bash completion script (in contrib) are aware of more commands and |
||||
options. |
||||
|
||||
* You can be warned when core.autocrlf conversion is applied in |
||||
such a way that results in an irreversible conversion. |
||||
|
||||
* A catch-all "color.ui" configuration variable can be used to |
||||
enable coloring of all color-capable commands, instead of |
||||
individual ones such as "color.status" and "color.branch". |
||||
|
||||
* The commands refused to take absolute pathnames where they |
||||
require pathnames relative to the work tree or the current |
||||
subdirectory. They now can take absolute pathnames in such a |
||||
case as long as the pathnames do not refer outside of the |
||||
work tree. E.g. "git add $(pwd)/foo" now works. |
||||
|
||||
* Error messages used to be sent to stderr, only to get hidden, |
||||
when $PAGER was in use. They now are sent to stdout along |
||||
with the command output to be shown in the $PAGER. |
||||
|
||||
* A pattern "foo/" in .gitignore file now matches a directory |
||||
"foo". Pattern "foo" also matches as before. |
||||
|
||||
* bash completion's prompt helper function can talk about |
||||
operation in-progress (e.g. merge, rebase, etc.). |
||||
|
||||
* Configuration variables "url.<usethis>.insteadof = <otherurl>" can be |
||||
used to tell "git-fetch" and "git-push" to use different URL than what |
||||
is given from the command line. |
||||
|
||||
* "git add -i" behaves better even before you make an initial commit. |
||||
|
||||
* "git am" refused to run from a subdirectory without a good reason. |
||||
|
||||
* After "git apply --whitespace=fix" fixes whitespace errors in a patch, |
||||
a line before the fix can appear as a context or preimage line in a |
||||
later patch, causing the patch not to apply. The command now knows to |
||||
see through whitespace fixes done to context lines to successfully |
||||
apply such a patch series. |
||||
|
||||
* "git branch" (and "git checkout -b") to branch from a local branch can |
||||
optionally set "branch.<name>.merge" to mark the new branch to build on |
||||
the other local branch, when "branch.autosetupmerge" is set to |
||||
"always", or when passing the command line option "--track" (this option |
||||
was ignored when branching from local branches). By default, this does |
||||
not happen when branching from a local branch. |
||||
|
||||
* "git checkout" to switch to a branch that has "branch.<name>.merge" set |
||||
(i.e. marked to build on another branch) reports how much the branch |
||||
and the other branch diverged. |
||||
|
||||
* When "git checkout" has to update a lot of paths, it used to be silent |
||||
for 4 seconds before it showed any progress report. It is now a bit |
||||
more impatient and starts showing progress report early. |
||||
|
||||
* "git commit" learned a new hook "prepare-commit-msg" that can |
||||
inspect what is going to be committed and prepare the commit |
||||
log message template to be edited. |
||||
|
||||
* "git cvsimport" can now take more than one -M options. |
||||
|
||||
* "git describe" learned to limit the tags to be used for |
||||
naming with --match option. |
||||
|
||||
* "git describe --contains" now barfs when the named commit |
||||
cannot be described. |
||||
|
||||
* "git describe --exact-match" describes only commits that are tagged. |
||||
|
||||
* "git describe --long" describes a tagged commit as $tag-0-$sha1, |
||||
instead of just showing the exact tagname. |
||||
|
||||
* "git describe" warns when using a tag whose name and path contradict |
||||
with each other. |
||||
|
||||
* "git diff" learned "--relative" option to limit and output paths |
||||
relative to the current directory when working in a subdirectory. |
||||
|
||||
* "git diff" learned "--dirstat" option to show birds-eye-summary of |
||||
changes more concisely than "--diffstat". |
||||
|
||||
* "git format-patch" learned --cover-letter option to generate a cover |
||||
letter template. |
||||
|
||||
* "git gc" learned --quiet option. |
||||
|
||||
* "git gc" now automatically prunes unreachable objects that are two |
||||
weeks old or older. |
||||
|
||||
* "git gc --auto" can be disabled more easily by just setting gc.auto |
||||
to zero. It also tolerates more packfiles by default. |
||||
|
||||
* "git grep" now knows "--name-only" is a synonym for the "-l" option. |
||||
|
||||
* "git help <alias>" now reports "'git <alias>' is alias to <what>", |
||||
instead of saying "No manual entry for git-<alias>". |
||||
|
||||
* "git help" can use different backends to show manual pages and this can |
||||
be configured using "man.viewer" configuration. |
||||
|
||||
* "gitk" does not restore window position from $HOME/.gitk anymore (it |
||||
still restores the size). |
||||
|
||||
* "git log --grep=<what>" learned "--fixed-strings" option to look for |
||||
<what> without treating it as a regular expression. |
||||
|
||||
* "git gui" learned an auto-spell checking. |
||||
|
||||
* "git push <somewhere> HEAD" and "git push <somewhere> +HEAD" works as |
||||
expected; they push the current branch (and only the current branch). |
||||
In addition, HEAD can be written as the value of "remote.<there>.push" |
||||
configuration variable. |
||||
|
||||
* When the configuration variable "pack.threads" is set to 0, "git |
||||
repack" auto detects the number of CPUs and uses that many threads. |
||||
|
||||
* "git send-email" learned to prompt for passwords |
||||
interactively. |
||||
|
||||
* "git send-email" learned an easier way to suppress CC |
||||
recipients. |
||||
|
||||
* "git stash" learned "pop" command, that applies the latest stash and |
||||
removes it from the stash, and "drop" command to discard the named |
||||
stash entry. |
||||
|
||||
* "git submodule" learned a new subcommand "summary" to show the |
||||
symmetric difference between the HEAD version and the work tree version |
||||
of the submodule commits. |
||||
|
||||
* Various "git cvsimport", "git cvsexportcommit", "git cvsserver", |
||||
"git svn" and "git p4" improvements. |
||||
|
||||
(internal) |
||||
|
||||
* Duplicated code between git-help and git-instaweb that |
||||
launches user's preferred browser has been refactored. |
||||
|
||||
* It is now easier to write test scripts that records known |
||||
breakages. |
||||
|
||||
* "git checkout" is rewritten in C. |
||||
|
||||
* "git remote" is rewritten in C. |
||||
|
||||
* Two conflict hunks that are separated by a very short span of common |
||||
lines are now coalesced into one larger hunk, to make the result easier |
||||
to read. |
||||
|
||||
* Run-command API's use of file descriptors is documented clearer and |
||||
is more consistent now. |
||||
|
||||
* diff output can be sent to FILE * that is different from stdout. This |
||||
will help reimplementing more things in C. |
||||
|
||||
Fixes since v1.5.4 |
||||
------------------ |
||||
|
||||
All of the fixes in v1.5.4 maintenance series are included in |
||||
this release, unless otherwise noted. |
||||
|
||||
* "git-http-push" did not allow deletion of remote ref with the usual |
||||
"push <remote> :<branch>" syntax. |
||||
|
||||
* "git-rebase --abort" did not go back to the right location if |
||||
"git-reset" was run during the "git-rebase" session. |
||||
|
||||
* "git imap-send" without setting imap.host did not error out but |
||||
segfaulted. |
@ -0,0 +1,28 @@
@@ -0,0 +1,28 @@
|
||||
GIT v1.5.6.1 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.6 |
||||
------------------ |
||||
|
||||
* Last minute change broke loose object creation on AIX. |
||||
|
||||
* (performance fix) We used to make $GIT_DIR absolute path early in the |
||||
programs but keeping it relative to the current directory internally |
||||
gives 1-3 per-cent performance boost. |
||||
|
||||
* bash completion knows the new --graph option to git-log family. |
||||
|
||||
|
||||
* git-diff -c/--cc showed unnecessary "deletion" lines at the context |
||||
boundary. |
||||
|
||||
* git-for-each-ref ignored %(object) and %(type) requests for tag |
||||
objects. |
||||
|
||||
* git-merge usage had a typo. |
||||
|
||||
* Rebuilding of git-svn metainfo database did not take rewriteRoot |
||||
option into account. |
||||
|
||||
* Running "git-rebase --continue/--skip/--abort" before starting a |
||||
rebase gave nonsense error messages. |
@ -0,0 +1,40 @@
@@ -0,0 +1,40 @@
|
||||
GIT v1.5.6.2 Release Notes |
||||
========================== |
||||
|
||||
Futureproof |
||||
----------- |
||||
|
||||
* "git-shell" accepts requests without a dash between "git" and |
||||
subcommand name (e.g. "git upload-pack") which the newer client will |
||||
start to make sometime in the future. |
||||
|
||||
Fixes since v1.5.6.1 |
||||
-------------------- |
||||
|
||||
* "git clone" from a remote that is named with url.insteadOf setting in |
||||
$HOME/.gitconfig did not work well. |
||||
|
||||
* "git describe --long --tags" segfaulted when the described revision was |
||||
tagged with a lightweight tag. |
||||
|
||||
* "git diff --check" did not report the result via its exit status |
||||
reliably. |
||||
|
||||
* When remote side used to have branch 'foo' and git-fetch finds that now |
||||
it has branch 'foo/bar', it refuses to lose the existing remote tracking |
||||
branch and its reflog. The error message has been improved to suggest |
||||
pruning the remote if the user wants to proceed and get the latest set |
||||
of branches from the remote, including such 'foo/bar'. |
||||
|
||||
* "git reset file" should mean the same thing as "git reset HEAD file", |
||||
but we required disambiguating -- even when "file" is not ambiguous. |
||||
|
||||
* "git show" segfaulted when an annotated tag that points at another |
||||
annotated tag was given to it. |
||||
|
||||
* Optimization for a large import via "git-svn" introduced in v1.5.6 had a |
||||
serious memory and temporary file leak, which made it unusable for |
||||
moderately large import. |
||||
|
||||
* "git-svn" mangled remote nickname used in the configuration file |
||||
unnecessarily. |
@ -0,0 +1,52 @@
@@ -0,0 +1,52 @@
|
||||
GIT v1.5.6.3 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.6.2 |
||||
-------------------- |
||||
|
||||
* Setting core.sharerepository to traditional "true" value was supposed to make |
||||
the repository group writable but should not affect permission for others. |
||||
However, since 1.5.6, it was broken to drop permission for others when umask is |
||||
022, making the repository unreadable by others. |
||||
|
||||
* Setting GIT_TRACE will report spawning of external process via run_command(). |
||||
|
||||
* Using an object with very deep delta chain pinned memory needed for extracting |
||||
intermediate base objects unnecessarily long, leading to excess memory usage. |
||||
|
||||
* Bash completion script did not notice '--' marker on the command |
||||
line and tried the relatively slow "ref completion" even when |
||||
completing arguments after one. |
||||
|
||||
* Registering a non-empty blob racily and then truncating the working |
||||
tree file for it confused "racy-git avoidance" logic into thinking |
||||
that the path is now unchanged. |
||||
|
||||
* The section that describes attributes related to git-archive were placed |
||||
in a wrong place in the gitattributes(5) manual page. |
||||
|
||||
* "git am" was not helpful to the users when it detected that the committer |
||||
information is not set up properly yet. |
||||
|
||||
* "git clone" had a leftover debugging fprintf(). |
||||
|
||||
* "git clone -q" was not quiet enough as it used to and gave object count |
||||
and progress reports. |
||||
|
||||
* "git clone" marked downloaded packfile with .keep; this could be a |
||||
good thing if the remote side is well packed but otherwise not, |
||||
especially for a project that is not really big. |
||||
|
||||
* "git daemon" used to call syslog() from a signal handler, which |
||||
could raise signals of its own but generally is not reentrant. This |
||||
was fixed by restructuring the code to report syslog() after the handler |
||||
returns. |
||||
|
||||
* When "git push" tries to remove a remote ref, and corresponding |
||||
tracking ref is missing, we used to report error (i.e. failure to |
||||
remove something that does not exist). |
||||
|
||||
* "git mailinfo" (hence "git am") did not handle commit log messages in a |
||||
MIME multipart mail correctly. |
||||
|
||||
Contains other various documentation fixes. |
@ -0,0 +1,47 @@
@@ -0,0 +1,47 @@
|
||||
GIT v1.5.6.4 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.6.3 |
||||
-------------------- |
||||
|
||||
* Various commands could overflow its internal buffer on a platform |
||||
with small PATH_MAX value in a repository that has contents with |
||||
long pathnames. |
||||
|
||||
* There wasn't a way to make --pretty=format:%<> specifiers to honor |
||||
.mailmap name rewriting for authors and committers. Now you can with |
||||
%aN and %cN. |
||||
|
||||
* Bash completion wasted too many cycles; this has been optimized to be |
||||
usable again. |
||||
|
||||
* Bash completion lost ref part when completing something like "git show |
||||
pu:Makefile". |
||||
|
||||
* "git-cvsserver" did not clean up its temporary working area after annotate |
||||
request. |
||||
|
||||
* "git-daemon" called syslog() from its signal handler, which was a |
||||
no-no. |
||||
|
||||
* "git-fetch" into an empty repository used to remind that the fetch will |
||||
be huge by saying "no common commits", but this was an unnecessary |
||||
noise; it is already known by the user anyway. |
||||
|
||||
* "git-http-fetch" would have segfaulted when pack idx file retrieved |
||||
from the other side was corrupt. |
||||
|
||||
* "git-index-pack" used too much memory when dealing with a deep delta chain. |
||||
|
||||
* "git-mailinfo" (hence "git-am") did not correctly handle in-body [PATCH] |
||||
line to override the commit title taken from the mail Subject header. |
||||
|
||||
* "git-rebase -i -p" lost parents that are not involved in the history |
||||
being rewritten. |
||||
|
||||
* "git-rm" lost track of where the index file was when GIT_DIR was |
||||
specified as a relative path. |
||||
|
||||
* "git-rev-list --quiet" was not quiet as advertised. |
||||
|
||||
Contains other various documentation fixes. |
@ -0,0 +1,29 @@
@@ -0,0 +1,29 @@
|
||||
GIT v1.5.6.5 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.5.6.4 |
||||
-------------------- |
||||
|
||||
* "git cvsimport" used to spit out "UNKNOWN LINE..." diagnostics to stdout. |
||||
|
||||
* "git commit -F filename" and "git tag -F filename" run from subdirectories |
||||
did not read the right file. |
||||
|
||||
* "git init --template=" with blank "template" parameter linked files |
||||
under root directories to .git, which was a total nonsense. Instead, it |
||||
means "I do not want to use anything from the template directory". |
||||
|
||||
* "git diff-tree" and other diff plumbing ignored diff.renamelimit configuration |
||||
variable when the user explicitly asked for rename detection. |
||||
|
||||
* "git name-rev --name-only" did not work when "--stdin" option was in effect. |
||||
|
||||
* "git show-branch" mishandled its 8th branch. |
||||
|
||||
* Addition of "git update-index --ignore-submodules" that happened during |
||||
1.5.6 cycle broke "git update-index --ignore-missing". |
||||
|
||||
* "git send-email" did not parse charset from an existing Content-type: |
||||
header properly. |
||||
|
||||
Contains other various documentation fixes. |
@ -0,0 +1,10 @@
@@ -0,0 +1,10 @@
|
||||
GIT v1.5.6.6 Release Notes |
||||
========================== |
||||
|
||||
Fixes since 1.5.6.5 |
||||
------------------- |
||||
|
||||
* Removed support for an obsolete gitweb request URI, whose |
||||
implementation ran "git diff" Porcelain, instead of using plumbing, |
||||
which would have run an external diff command specified in the |
||||
repository configuration as the gitweb user. |
@ -0,0 +1,115 @@
@@ -0,0 +1,115 @@
|
||||
GIT v1.5.6 Release Notes |
||||
======================== |
||||
|
||||
Updates since v1.5.5 |
||||
-------------------- |
||||
|
||||
(subsystems) |
||||
|
||||
* Comes with updated gitk and git-gui. |
||||
|
||||
(portability) |
||||
|
||||
* git will build on AIX better than before now. |
||||
|
||||
* core.ignorecase configuration variable can be used to work better on |
||||
filesystems that are not case sensitive. |
||||
|
||||
* "git init" now autodetects the case sensitivity of the filesystem and |
||||
sets core.ignorecase accordingly. |
||||
|
||||
* cpio is no longer used; neither "curl" binary (libcurl is still used). |
||||
|
||||
(documentation) |
||||
|
||||
* Many freestanding documentation pages have been converted and made |
||||
available to "git help" (aka "man git<something>") as section 7 of |
||||
the manual pages. This means bookmarks to some HTML documentation |
||||
files may need to be updated (eg "tutorial.html" became |
||||
"gittutorial.html"). |
||||
|
||||
(performance) |
||||
|
||||
* "git clone" was rewritten in C. This will hopefully help cloning a |
||||
repository with insane number of refs. |
||||
|
||||
* "git rebase --onto $there $from $branch" used to switch to the tip of |
||||
$branch only to immediately reset back to $from, smudging work tree |
||||
files unnecessarily. This has been optimized. |
||||
|
||||
* Object creation codepath in "git-svn" has been optimized by enhancing |
||||
plumbing commands git-cat-file and git-hash-object. |
||||
|
||||
(usability, bells and whistles) |
||||
|
||||
* "git add -p" (and the "patch" subcommand of "git add -i") can choose to |
||||
apply (or not apply) mode changes independently from contents changes. |
||||
|
||||
* "git bisect help" gives longer and more helpful usage information. |
||||
|
||||
* "git bisect" does not use a special branch "bisect" anymore; instead, it |
||||
does its work on a detached HEAD. |
||||
|
||||
* "git branch" (and "git checkout -b") can be told to set up |
||||
branch.<name>.rebase automatically, so that later you can say "git pull" |
||||
and magically cause "git pull --rebase" to happen. |
||||
|
||||
* "git branch --merged" and "git branch --no-merged" can be used to list |
||||
branches that have already been merged (or not yet merged) to the |
||||
current branch. |
||||
|
||||
* "git cherry-pick" and "git revert" can add a sign-off. |
||||
|
||||
* "git commit" mentions the author identity when you are committing |
||||
somebody else's changes. |
||||
|
||||
* "git diff/log --dirstat" output is consistent between binary and textual |
||||
changes. |
||||
|
||||
* "git filter-branch" rewrites signed tags by demoting them to annotated. |
||||
|
||||
* "git format-patch --no-binary" can produce a patch that lack binary |
||||
changes (i.e. cannot be used to propagate the whole changes) meant only |
||||
for reviewing. |
||||
|
||||
* "git init --bare" is a synonym for "git --bare init" now. |
||||
|
||||
* "git gc --auto" honors a new pre-auto-gc hook to temporarily disable it. |
||||
|
||||
* "git log --pretty=tformat:<custom format>" gives a LF after each entry, |
||||
instead of giving a LF between each pair of entries which is how |
||||
"git log --pretty=format:<custom format>" works. |
||||
|
||||
* "git log" and friends learned the "--graph" option to show the ancestry |
||||
graph at the left margin of the output. |
||||
|
||||
* "git log" and friends can be told to use date format that is different |
||||
from the default via 'log.date' configuration variable. |
||||
|
||||
* "git send-email" now can send out messages outside a git repository. |
||||
|
||||
* "git send-email --compose" was made aware of rfc2047 quoting. |
||||
|
||||
* "git status" can optionally include output from "git submodule |
||||
summary". |
||||
|
||||
* "git svn" learned --add-author-from option to propagate the authorship |
||||
by munging the commit log message. |
||||
|
||||
* new object creation and looking up in "git svn" has been optimized. |
||||
|
||||
* "gitweb" can read from a system-wide configuration file. |
||||
|
||||
(internal) |
||||
|
||||
* "git unpack-objects" and "git receive-pack" is now more strict about |
||||
detecting breakage in the objects they receive over the wire. |
||||
|
||||
|
||||
Fixes since v1.5.5 |
||||
------------------ |
||||
|
||||
All of the fixes in v1.5.5 maintenance series are included in |
||||
this release, unless otherwise noted. |
||||
|
||||
And there are too numerous small fixes to otherwise note here ;-) |
@ -0,0 +1,36 @@
@@ -0,0 +1,36 @@
|
||||
GIT v1.6.0.1 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.0 |
||||
------------------ |
||||
|
||||
* "git diff --cc" did not honor content mangling specified by |
||||
gitattributes and core.autocrlf when reading from the work tree. |
||||
|
||||
* "git diff --check" incorrectly detected new trailing blank lines when |
||||
whitespace check was in effect. |
||||
|
||||
* "git for-each-ref" tried to dereference NULL when asked for '%(body)" on |
||||
a tag with a single incomplete line as its payload. |
||||
|
||||
* "git format-patch" peeked before the beginning of a string when |
||||
"format.headers" variable is empty (a misconfiguration). |
||||
|
||||
* "git help help" did not work correctly. |
||||
|
||||
* "git mailinfo" (hence "git am") was unhappy when MIME multipart message |
||||
contained garbage after the finishing boundary. |
||||
|
||||
* "git mailinfo" also was unhappy when the "From: " line only had a bare |
||||
e-mail address. |
||||
|
||||
* "git merge" did not refresh the index correctly when a merge resulted in |
||||
a fast-forward. |
||||
|
||||
* "git merge" did not resolve a truly trivial merges that can be done |
||||
without content level merges. |
||||
|
||||
* "git svn dcommit" to a repository with URL that has embedded usernames |
||||
did not work correctly. |
||||
|
||||
Contains other various documentation fixes. |
@ -0,0 +1,87 @@
@@ -0,0 +1,87 @@
|
||||
GIT v1.6.0.2 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.0.1 |
||||
-------------------- |
||||
|
||||
* Installation on platforms that needs .exe suffix to git-* programs were |
||||
broken in 1.6.0.1. |
||||
|
||||
* Installation on filesystems without symbolic links support did not |
||||
work well. |
||||
|
||||
* In-tree documentations and test scripts now use "git foo" form to set a |
||||
better example, instead of the "git-foo" form (which is an acceptable |
||||
form if you have "PATH=$(git --exec-path):$PATH" in your script) |
||||
|
||||
* Many commands did not use the correct working tree location when used |
||||
with GIT_WORK_TREE environment settings. |
||||
|
||||
* Some systems needs to use compatibility fnmach and regex libraries |
||||
independent from each other; the compat/ area has been reorganized to |
||||
allow this. |
||||
|
||||
|
||||
* "git apply --unidiff-zero" incorrectly applied a -U0 patch that inserts |
||||
a new line before the second line. |
||||
|
||||
* "git blame -c" did not exactly work like "git annotate" when range |
||||
boundaries are involved. |
||||
|
||||
* "git checkout file" when file is still unmerged checked out contents from |
||||
a random high order stage, which was confusing. |
||||
|
||||
* "git clone $there $here/" with extra trailing slashes after explicit |
||||
local directory name $here did not work as expected. |
||||
|
||||
* "git diff" on tracked contents with CRLF line endings did not drive "less" |
||||
intelligently when showing added or removed lines. |
||||
|
||||
* "git diff --dirstat -M" did not add changes in subdirectories up |
||||
correctly for renamed paths. |
||||
|
||||
* "git diff --cumulative" did not imply "--dirstat". |
||||
|
||||
* "git for-each-ref refs/heads/" did not work as expected. |
||||
|
||||
* "git gui" allowed users to feed patch without any context to be applied. |
||||
|
||||
* "git gui" botched parsing "diff" output when a line that begins with two |
||||
dashes and a space gets removed or a line that begins with two pluses |
||||
and a space gets added. |
||||
|
||||
* "git gui" translation updates and i18n fixes. |
||||
|
||||
* "git index-pack" is more careful against disk corruption while completing |
||||
a thin pack. |
||||
|
||||
* "git log -i --grep=pattern" did not ignore case; neither "git log -E |
||||
--grep=pattern" triggered extended regexp. |
||||
|
||||
* "git log --pretty="%ad" --date=short" did not use short format when |
||||
showing the timestamp. |
||||
|
||||
* "git log --author=author" match incorrectly matched with the |
||||
timestamp part of "author " line in commit objects. |
||||
|
||||
* "git log -F --author=author" did not work at all. |
||||
|
||||
* Build procedure for "git shell" that used stub versions of some |
||||
functions and globals was not understood by linkers on some platforms. |
||||
|
||||
* "git stash" was fooled by a stat-dirty but otherwise unmodified paths |
||||
and refused to work until the user refreshed the index. |
||||
|
||||
* "git svn" was broken on Perl before 5.8 with recent fixes to reduce |
||||
use of temporary files. |
||||
|
||||
* "git verify-pack -v" did not work correctly when given more than one |
||||
packfile. |
||||
|
||||
Also contains many documentation updates. |
||||
|
||||
-- |
||||
exec >/var/tmp/1 |
||||
O=v1.6.0.1-78-g3632cfc |
||||
echo O=$(git describe maint) |
||||
git shortlog --no-merges $O..maint |
@ -0,0 +1,117 @@
@@ -0,0 +1,117 @@
|
||||
GIT v1.6.0.3 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.0.2 |
||||
-------------------- |
||||
|
||||
* "git archive --format=zip" did not honor core.autocrlf while |
||||
--format=tar did. |
||||
|
||||
* Continuing "git rebase -i" was very confused when the user left modified |
||||
files in the working tree while resolving conflicts. |
||||
|
||||
* Continuing "git rebase -i" was also very confused when the user left |
||||
some staged changes in the index after "edit". |
||||
|
||||
* "git rebase -i" now honors the pre-rebase hook, just like the |
||||
other rebase implementations "git rebase" and "git rebase -m". |
||||
|
||||
* "git rebase -i" incorrectly aborted when there is no commit to replay. |
||||
|
||||
* Behaviour of "git diff --quiet" was inconsistent with "diff --exit-code" |
||||
with the output redirected to /dev/null. |
||||
|
||||
* "git diff --no-index" on binary files no longer outputs a bogus |
||||
"diff --git" header line. |
||||
|
||||
* "git diff" hunk header patterns with multiple elements separated by LF |
||||
were not used correctly. |
||||
|
||||
* Hunk headers in "git diff" default to using extended regular |
||||
expressions, fixing some of the internal patterns on non-GNU |
||||
platforms. |
||||
|
||||
* New config "diff.*.xfuncname" exposes extended regular expressions |
||||
for user specified hunk header patterns. |
||||
|
||||
* "git gc" when ejecting otherwise unreachable objects from packfiles into |
||||
loose form leaked memory. |
||||
|
||||
* "git index-pack" was recently broken and mishandled objects added by |
||||
thin-pack completion processing under memory pressure. |
||||
|
||||
* "git index-pack" was recently broken and misbehaved when run from inside |
||||
.git/objects/pack/ directory. |
||||
|
||||
* "git stash apply sash@{1}" was fixed to error out. Prior versions |
||||
would have applied stash@{0} incorrectly. |
||||
|
||||
* "git stash apply" now offers a better suggestion on how to continue |
||||
if the working tree is currently dirty. |
||||
|
||||
* "git for-each-ref --format=%(subject)" fixed for commits with no |
||||
no newline in the message body. |
||||
|
||||
* "git remote" fixed to protect printf from user input. |
||||
|
||||
* "git remote show -v" now displays all URLs of a remote. |
||||
|
||||
* "git checkout -b branch" was confused when branch already existed. |
||||
|
||||
* "git checkout -q" once again suppresses the locally modified file list. |
||||
|
||||
* "git clone -q", "git fetch -q" asks remote side to not send |
||||
progress messages, actually making their output quiet. |
||||
|
||||
* Cross-directory renames are no longer used when creating packs. This |
||||
allows more graceful behavior on filesystems like sshfs. |
||||
|
||||
* Stale temporary files under $GIT_DIR/objects/pack are now cleaned up |
||||
automatically by "git prune". |
||||
|
||||
* "git merge" once again removes directories after the last file has |
||||
been removed from it during the merge. |
||||
|
||||
* "git merge" did not allocate enough memory for the structure itself when |
||||
enumerating the parents of the resulting commit. |
||||
|
||||
* "git blame -C -C" no longer segfaults while trying to pass blame if |
||||
it encounters a submodule reference. |
||||
|
||||
* "git rm" incorrectly claimed that you have local modifications when a |
||||
path was merely stat-dirty. |
||||
|
||||
* "git svn" fixed to display an error message when 'set-tree' failed, |
||||
instead of a Perl compile error. |
||||
|
||||
* "git submodule" fixed to handle checking out a different commit |
||||
than HEAD after initializing the submodule. |
||||
|
||||
* The "git commit" error message when there are still unmerged |
||||
files present was clarified to match "git write-tree". |
||||
|
||||
* "git init" was confused when core.bare or core.sharedRepository are set |
||||
in system or user global configuration file by mistake. When --bare or |
||||
--shared is given from the command line, these now override such |
||||
settings made outside the repositories. |
||||
|
||||
* Some segfaults due to uncaught NULL pointers were fixed in multiple |
||||
tools such as apply, reset, update-index. |
||||
|
||||
* Solaris builds now default to OLD_ICONV=1 to avoid compile warnings; |
||||
Solaris 8 does not define NEEDS_LIBICONV by default. |
||||
|
||||
* "Git.pm" tests relied on unnecessarily more recent version of Perl. |
||||
|
||||
* "gitweb" triggered undef warning on commits without log messages. |
||||
|
||||
* "gitweb" triggered undef warnings on missing trees. |
||||
|
||||
* "gitweb" now removes PATH_INFO from its URLs so users don't have |
||||
to manually set the URL in the gitweb configuration. |
||||
|
||||
* Bash completion removed support for legacy "git-fetch", "git-push" |
||||
and "git-pull" as these are no longer installed. Dashless form |
||||
("git fetch") is still however supported. |
||||
|
||||
Many other documentation updates. |
@ -0,0 +1,39 @@
@@ -0,0 +1,39 @@
|
||||
GIT v1.6.0.4 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.0.3 |
||||
-------------------- |
||||
|
||||
* 'git add -p' said "No changes" when only binary files were changed. |
||||
|
||||
* 'git archive' did not work correctly in bare repositories. |
||||
|
||||
* 'git checkout -t -b newbranch' when you are on detached HEAD was broken. |
||||
|
||||
* when we refuse to detect renames because there are too many new or |
||||
deleted files, 'git diff' did not say how many there are. |
||||
|
||||
* 'git push --mirror' tried and failed to push the stash; there is no |
||||
point in sending it to begin with. |
||||
|
||||
* 'git push' did not update the remote tracking reference if the corresponding |
||||
ref on the remote end happened to be already up to date. |
||||
|
||||
* 'git pull $there $branch:$current_branch' did not work when you were on |
||||
a branch yet to be born. |
||||
|
||||
* when giving up resolving a conflicted merge, 'git reset --hard' failed |
||||
to remove new paths from the working tree. |
||||
|
||||
* 'git send-email' had a small fd leak while scanning directory. |
||||
|
||||
* 'git status' incorrectly reported a submodule directory as an untracked |
||||
directory. |
||||
|
||||
* 'git svn' used deprecated 'git-foo' form of subcommand invocation. |
||||
|
||||
* 'git update-ref -d' to remove a reference did not honor --no-deref option. |
||||
|
||||
* Plugged small memleaks here and there. |
||||
|
||||
* Also contains many documentation updates. |
@ -0,0 +1,56 @@
@@ -0,0 +1,56 @@
|
||||
GIT v1.6.0.5 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.0.4 |
||||
-------------------- |
||||
|
||||
* "git checkout" used to crash when your HEAD was pointing at a deleted |
||||
branch. |
||||
|
||||
* "git checkout" from an un-checked-out state did not allow switching out |
||||
of the current branch. |
||||
|
||||
* "git diff" always allowed GIT_EXTERNAL_DIFF and --no-ext-diff was no-op for |
||||
the command. |
||||
|
||||
* Giving 3 or more tree-ish to "git diff" is supposed to show the combined |
||||
diff from second and subsequent trees to the first one, but the order was |
||||
screwed up. |
||||
|
||||
* "git fast-export" did not export all tags. |
||||
|
||||
* "git ls-files --with-tree=<tree>" did not work with options other |
||||
than -c, most notably with -m. |
||||
|
||||
* "git pack-objects" did not make its best effort to honor --max-pack-size |
||||
option when a single first object already busted the given limit and |
||||
placed many objects in a single pack. |
||||
|
||||
* "git-p4" fast import frontend was too eager to trigger its keyword expansion |
||||
logic, even on a keyword-looking string that does not have closing '$' on the |
||||
same line. |
||||
|
||||
* "git push $there" when the remote $there is defined in $GIT_DIR/branches/$there |
||||
behaves more like what cg-push from Cogito used to work. |
||||
|
||||
* when giving up resolving a conflicted merge, "git reset --hard" failed |
||||
to remove new paths from the working tree. |
||||
|
||||
* "git tag" did not complain when given mutually incompatible set of options. |
||||
|
||||
* The message constructed in the internal editor was discarded when "git |
||||
tag -s" failed to sign the message, which was often caused by the user |
||||
not configuring GPG correctly. |
||||
|
||||
* "make check" cannot be run without sparse; people may have meant to say |
||||
"make test" instead, so suggest that. |
||||
|
||||
* Internal diff machinery had a corner case performance bug that choked on |
||||
a large file with many repeated contents. |
||||
|
||||
* "git repack" used to grab objects out of packs marked with .keep |
||||
into a new pack. |
||||
|
||||
* Many unsafe call to sprintf() style varargs functions are corrected. |
||||
|
||||
* Also contains quite a few documentation updates. |
@ -0,0 +1,33 @@
@@ -0,0 +1,33 @@
|
||||
GIT v1.6.0.6 Release Notes |
||||
========================== |
||||
|
||||
Fixes since 1.6.0.5 |
||||
------------------- |
||||
|
||||
* "git fsck" had a deep recursion that wasted stack space. |
||||
|
||||
* "git fast-export" and "git fast-import" choked on an old style |
||||
annotated tag that lack the tagger information. |
||||
|
||||
* "git mergetool -- file" did not correctly skip "--" marker that |
||||
signals the end of options list. |
||||
|
||||
* "git show $tag" segfaulted when an annotated $tag pointed at a |
||||
nonexistent object. |
||||
|
||||
* "git show 2>error" when the standard output is automatically redirected |
||||
to the pager redirected the standard error to the pager as well; there |
||||
was no need to. |
||||
|
||||
* "git send-email" did not correctly handle list of addresses when |
||||
they had quoted comma (e.g. "Lastname, Givenname" <mail@addre.ss>). |
||||
|
||||
* Logic to discover branch ancestry in "git svn" was unreliable when |
||||
the process to fetch history was interrupted. |
||||
|
||||
* Removed support for an obsolete gitweb request URI, whose |
||||
implementation ran "git diff" Porcelain, instead of using plumbing, |
||||
which would have run an external diff command specified in the |
||||
repository configuration as the gitweb user. |
||||
|
||||
Also contains numerous documentation typofixes. |
@ -0,0 +1,258 @@
@@ -0,0 +1,258 @@
|
||||
GIT v1.6.0 Release Notes |
||||
======================== |
||||
|
||||
User visible changes |
||||
-------------------- |
||||
|
||||
With the default Makefile settings, most of the programs are now |
||||
installed outside your $PATH, except for "git", "gitk" and |
||||
some server side programs that need to be accessible for technical |
||||
reasons. Invoking a git subcommand as "git-xyzzy" from the command |
||||
line has been deprecated since early 2006 (and officially announced in |
||||
1.5.4 release notes); use of them from your scripts after adding |
||||
output from "git --exec-path" to the $PATH is still supported in this |
||||
release, but users are again strongly encouraged to adjust their |
||||
scripts to use "git xyzzy" form, as we will stop installing |
||||
"git-xyzzy" hardlinks for built-in commands in later releases. |
||||
|
||||
An earlier change to page "git status" output was overwhelmingly unpopular |
||||
and has been reverted. |
||||
|
||||
Source changes needed for porting to MinGW environment are now all in the |
||||
main git.git codebase. |
||||
|
||||
By default, packfiles created with this version uses delta-base-offset |
||||
encoding introduced in v1.4.4. Pack idx files are using version 2 that |
||||
allows larger packs and added robustness thanks to its CRC checking, |
||||
introduced in v1.5.2 and v1.4.4.5. If you want to keep your repositories |
||||
backwards compatible past these versions, set repack.useDeltaBaseOffset |
||||
to false or pack.indexVersion to 1, respectively. |
||||
|
||||
We used to prevent sample hook scripts shipped in templates/ from |
||||
triggering by default by relying on the fact that we install them as |
||||
unexecutable, but on some filesystems, this approach does not work. |
||||
They are now shipped with ".sample" suffix. If you want to activate |
||||
any of these samples as-is, rename them to drop the ".sample" suffix, |
||||
instead of running "chmod +x" on them. For example, you can rename |
||||
hooks/post-update.sample to hooks/post-update to enable the sample |
||||
hook that runs update-server-info, in order to make repositories |
||||
friendly to dumb protocols (i.e. HTTP). |
||||
|
||||
GIT_CONFIG, which was only documented as affecting "git config", but |
||||
actually affected all git commands, now only affects "git config". |
||||
GIT_LOCAL_CONFIG, also only documented as affecting "git config" and |
||||
not different from GIT_CONFIG in a useful way, is removed. |
||||
|
||||
The ".dotest" temporary area "git am" and "git rebase" use is now moved |
||||
inside the $GIT_DIR, to avoid mistakes of adding it to the project by |
||||
accident. |
||||
|
||||
An ancient merge strategy "stupid" has been removed. |
||||
|
||||
|
||||
Updates since v1.5.6 |
||||
-------------------- |
||||
|
||||
(subsystems) |
||||
|
||||
* git-p4 in contrib learned "allowSubmit" configuration to control on |
||||
which branch to allow "submit" subcommand. |
||||
|
||||
* git-gui learned to stage changes per-line. |
||||
|
||||
(portability) |
||||
|
||||
* Changes for MinGW port have been merged, thanks to Johannes Sixt and |
||||
gangs. |
||||
|
||||
* Sample hook scripts shipped in templates/ are now suffixed with |
||||
*.sample. |
||||
|
||||
* perl's in-place edit (-i) does not work well without backup files on Windows; |
||||
some tests are rewritten to cope with this. |
||||
|
||||
(documentation) |
||||
|
||||
* Updated howto/update-hook-example |
||||
|
||||
* Got rid of usage of "git-foo" from the tutorial and made typography |
||||
more consistent. |
||||
|
||||
* Disambiguating "--" between revs and paths is finally documented. |
||||
|
||||
(performance, robustness, sanity etc.) |
||||
|
||||
* index-pack used too much memory when dealing with a deep delta chain. |
||||
This has been optimized. |
||||
|
||||
* reduced excessive inlining to shrink size of the "git" binary. |
||||
|
||||
* verify-pack checks the object CRC when using version 2 idx files. |
||||
|
||||
* When an object is corrupt in a pack, the object became unusable even |
||||
when the same object is available in a loose form, We now try harder to |
||||
fall back to these redundant objects when able. In particular, "git |
||||
repack -a -f" can be used to fix such a corruption as long as necessary |
||||
objects are available. |
||||
|
||||
* Performance of "git-blame -C -C" operation is vastly improved. |
||||
|
||||
* git-clone does not create refs in loose form anymore (it behaves as |
||||
if you immediately ran git-pack-refs after cloning). This will help |
||||
repositories with insanely large number of refs. |
||||
|
||||
* core.fsyncobjectfiles configuration can be used to ensure that the loose |
||||
objects created will be fsync'ed (this is only useful on filesystems |
||||
that does not order data writes properly). |
||||
|
||||
* "git commit-tree" plumbing can make Octopus with more than 16 parents. |
||||
"git commit" has been capable of this for quite some time. |
||||
|
||||
(usability, bells and whistles) |
||||
|
||||
* even more documentation pages are now accessible via "man" and "git help". |
||||
|
||||
* A new environment variable GIT_CEILING_DIRECTORIES can be used to stop |
||||
the discovery process of the toplevel of working tree; this may be useful |
||||
when you are working in a slow network disk and are outside any working tree, |
||||
as bash-completion and "git help" may still need to run in these places. |
||||
|
||||
* By default, stash entries never expire. Set reflogexpire in [gc |
||||
"refs/stash"] to a reasonable value to get traditional auto-expiration |
||||
behaviour back |
||||
|
||||
* Longstanding latency issue with bash completion script has been |
||||
addressed. This will need to be backmerged to 'maint' later. |
||||
|
||||
* pager.<cmd> configuration variable can be used to enable/disable the |
||||
default paging behaviour per command. |
||||
|
||||
* "git-add -i" has a new action 'e/dit' to allow you edit the patch hunk |
||||
manually. |
||||
|
||||
* git-am records the original tip of the branch in ORIG_HEAD before it |
||||
starts applying patches. |
||||
|
||||
* git-apply can handle a patch that touches the same path more than once |
||||
much better than before. |
||||
|
||||
* git-apply can be told not to trust the line counts recorded in the input |
||||
patch but recount, with the new --recount option. |
||||
|
||||
* git-apply can be told to apply a patch to a path deeper than what the |
||||
patch records with --directory option. |
||||
|
||||
* git-archive can be told to omit certain paths from its output using |
||||
export-ignore attributes. |
||||
|
||||
* git-archive uses the zlib default compression level when creating |
||||
zip archive. |
||||
|
||||
* git-archive's command line options --exec and --remote can take their |
||||
parameters as separate command line arguments, similar to other commands. |
||||
IOW, both "--exec=path" and "--exec path" are now supported. |
||||
|
||||
* With -v option, git-branch describes the remote tracking statistics |
||||
similar to the way git-checkout reports by how many commits your branch |
||||
is ahead/behind. |
||||
|
||||
* git-branch's --contains option used to always require a commit parameter |
||||
to limit the branches with; it now defaults to list branches that |
||||
contains HEAD if this parameter is omitted. |
||||
|
||||
* git-branch's --merged and --no-merged option used to always limit the |
||||
branches relative to the HEAD, but they can now take an optional commit |
||||
argument that is used in place of HEAD. |
||||
|
||||
* git-bundle can read the revision arguments from the standard input. |
||||
|
||||
* git-cherry-pick can replay a root commit now. |
||||
|
||||
* git-clone can clone from a remote whose URL would be rewritten by |
||||
configuration stored in $HOME/.gitconfig now. |
||||
|
||||
* "git-clone --mirror" is a handy way to set up a bare mirror repository. |
||||
|
||||
* git-cvsserver learned to respond to "cvs co -c". |
||||
|
||||
* git-diff --check now checks leftover merge conflict markers. |
||||
|
||||
* "git-diff -p" learned to grab a better hunk header lines in |
||||
BibTex, Pascal/Delphi, and Ruby files and also pays attention to |
||||
chapter and part boundary in TeX documents. |
||||
|
||||
* When remote side used to have branch 'foo' and git-fetch finds that now |
||||
it has branch 'foo/bar', it refuses to lose the existing remote tracking |
||||
branch and its reflog. The error message has been improved to suggest |
||||
pruning the remote if the user wants to proceed and get the latest set |
||||
of branches from the remote, including such 'foo/bar'. |
||||
|
||||
* fast-export learned to export and import marks file; this can be used to |
||||
interface with fast-import incrementally. |
||||
|
||||
* fast-import and fast-export learned to export and import gitlinks. |
||||
|
||||
* "gitk" left background process behind after being asked to dig very deep |
||||
history and the user killed the UI; the process is killed when the UI goes |
||||
away now. |
||||
|
||||
* git-rebase records the original tip of branch in ORIG_HEAD before it is |
||||
rewound. |
||||
|
||||
* "git rerere" can be told to update the index with auto-reused resolution |
||||
with rerere.autoupdate configuration variable. |
||||
|
||||
* git-rev-parse learned $commit^! and $commit^@ notations used in "log" |
||||
family. These notations are available in gitk as well, because the gitk |
||||
command internally uses rev-parse to interpret its arguments. |
||||
|
||||
* git-rev-list learned --children option to show child commits it |
||||
encountered during the traversal, instead of showing parent commits. |
||||
|
||||
* git-send-mail can talk not just over SSL but over TLS now. |
||||
|
||||
* git-shortlog honors custom output format specified with "--pretty=format:". |
||||
|
||||
* "git-stash save" learned --keep-index option. This lets you stash away the |
||||
local changes and bring the changes staged in the index to your working |
||||
tree for examination and testing. |
||||
|
||||
* git-stash also learned branch subcommand to create a new branch out of |
||||
stashed changes. |
||||
|
||||
* git-status gives the remote tracking statistics similar to the way |
||||
git-checkout reports by how many commits your branch is ahead/behind. |
||||
|
||||
* "git-svn dcommit" is now aware of auto-props setting the subversion user |
||||
has. |
||||
|
||||
* You can tell "git status -u" to even more aggressively omit checking |
||||
untracked files with --untracked-files=no. |
||||
|
||||
* Original SHA-1 value for "update-ref -d" is optional now. |
||||
|
||||
* Error codes from gitweb are made more descriptive where possible, rather |
||||
than "403 forbidden" as we used to issue everywhere. |
||||
|
||||
(internal) |
||||
|
||||
* git-merge has been reimplemented in C. |
||||
|
||||
|
||||
Fixes since v1.5.6 |
||||
------------------ |
||||
|
||||
All of the fixes in v1.5.6 maintenance series are included in |
||||
this release, unless otherwise noted. |
||||
|
||||
* git-clone ignored its -u option; the fix needs to be backported to |
||||
'maint'; |
||||
|
||||
* git-mv used to lose the distinction between changes that are staged |
||||
and that are only in the working tree, by staging both in the index |
||||
after moving such a path. |
||||
|
||||
* "git-rebase -i -p" rewrote the parents to wrong ones when amending |
||||
(either edit or squash) was involved, and did not work correctly |
||||
when fast forwarding. |
||||
|
@ -0,0 +1,59 @@
@@ -0,0 +1,59 @@
|
||||
GIT v1.6.1.1 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.1 |
||||
------------------ |
||||
|
||||
* "git add frotz/nitfol" when "frotz" is a submodule should have errored |
||||
out, but it didn't. |
||||
|
||||
* "git apply" took file modes from the patch text and updated the mode |
||||
bits of the target tree even when the patch was not about mode changes. |
||||
|
||||
* "git bisect view" on Cygwin did not launch gitk |
||||
|
||||
* "git checkout $tree" did not trigger an error. |
||||
|
||||
* "git commit" tried to remove COMMIT_EDITMSG from the work tree by mistake. |
||||
|
||||
* "git describe --all" complained when a commit is described with a tag, |
||||
which was nonsense. |
||||
|
||||
* "git diff --no-index --" did not trigger no-index (aka "use git-diff as |
||||
a replacement of diff on untracked files") behaviour. |
||||
|
||||
* "git format-patch -1 HEAD" on a root commit failed to produce patch |
||||
text. |
||||
|
||||
* "git fsck branch" did not work as advertised; instead it behaved the same |
||||
way as "git fsck". |
||||
|
||||
* "git log --pretty=format:%s" did not handle a multi-line subject the |
||||
same way as built-in log listers (i.e. shortlog, --pretty=oneline, etc.) |
||||
|
||||
* "git daemon", and "git merge-file" are more careful when freopen fails |
||||
and barf, instead of going on and writing to unopened filehandle. |
||||
|
||||
* "git http-push" did not like some RFC 4918 compliant DAV server |
||||
responses. |
||||
|
||||
* "git merge -s recursive" mistakenly overwritten an untracked file in the |
||||
work tree upon delete/modify conflict. |
||||
|
||||
* "git merge -s recursive" didn't leave the index unmerged for entries with |
||||
rename/delete conflicts. |
||||
|
||||
* "git merge -s recursive" clobbered untracked files in the work tree. |
||||
|
||||
* "git mv -k" with more than one erroneous paths misbehaved. |
||||
|
||||
* "git read-tree -m -u" hence branch switching incorrectly lost a |
||||
subdirectory in rare cases. |
||||
|
||||
* "git rebase -i" issued an unnecessary error message upon a user error of |
||||
marking the first commit to be "squash"ed. |
||||
|
||||
* "git shortlog" did not format a commit message with multi-line |
||||
subject correctly. |
||||
|
||||
Many documentation updates. |
@ -0,0 +1,39 @@
@@ -0,0 +1,39 @@
|
||||
GIT v1.6.1.2 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.1.1 |
||||
-------------------- |
||||
|
||||
* The logic for rename detection in internal diff used by commands like |
||||
"git diff" and "git blame" has been optimized to avoid loading the same |
||||
blob repeatedly. |
||||
|
||||
* We did not allow writing out a blob that is larger than 2GB for no good |
||||
reason. |
||||
|
||||
* "git format-patch -o $dir", when $dir is a relative directory, used it |
||||
as relative to the root of the work tree, not relative to the current |
||||
directory. |
||||
|
||||
* v1.6.1 introduced an optimization for "git push" into a repository (A) |
||||
that borrows its objects from another repository (B) to avoid sending |
||||
objects that are available in repository B, when they are not yet used |
||||
by repository A. However the code on the "git push" sender side was |
||||
buggy and did not work when repository B had new objects that are not |
||||
known by the sender. This caused pushing into a "forked" repository |
||||
served by v1.6.1 software using "git push" from v1.6.1 sometimes did not |
||||
work. The bug was purely on the "git push" sender side, and has been |
||||
corrected. |
||||
|
||||
* "git status -v" did not paint its diff output in colour even when |
||||
color.ui configuration was set. |
||||
|
||||
* "git ls-tree" learned --full-tree option to help Porcelain scripts that |
||||
want to always see the full path regardless of the current working |
||||
directory. |
||||
|
||||
* "git grep" incorrectly searched in work tree paths even when they are |
||||
marked as assume-unchanged. It now searches in the index entries. |
||||
|
||||
* "git gc" with no grace period needlessly ejected packed but unreachable |
||||
objects in their loose form, only to delete them right away. |
@ -0,0 +1,32 @@
@@ -0,0 +1,32 @@
|
||||
GIT v1.6.1.3 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.1.2 |
||||
-------------------- |
||||
|
||||
* "git diff --binary | git apply" pipeline did not work well when |
||||
a binary blob is changed to a symbolic link. |
||||
|
||||
* Some combinations of -b/-w/--ignore-space-at-eol to "git diff" did |
||||
not work as expected. |
||||
|
||||
* "git grep" did not pass the -I (ignore binary) option when |
||||
calling out an external grep program. |
||||
|
||||
* "git log" and friends include HEAD to the set of starting points |
||||
when --all is given. This makes a difference when you are not |
||||
on any branch. |
||||
|
||||
* "git mv" to move an untracked file to overwrite a tracked |
||||
contents misbehaved. |
||||
|
||||
* "git merge -s octopus" with many potential merge bases did not |
||||
work correctly. |
||||
|
||||
* RPM binary package installed the html manpages in a wrong place. |
||||
|
||||
Also includes minor documentation fixes and updates. |
||||
|
||||
|
||||
-- |
||||
git shortlog --no-merges v1.6.1.2-33-gc789350.. |
@ -0,0 +1,44 @@
@@ -0,0 +1,44 @@
|
||||
GIT v1.6.1.4 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.1.3 |
||||
-------------------- |
||||
|
||||
* .gitignore learned to handle backslash as a quoting mechanism for |
||||
comment introduction character "#". |
||||
This fix was first merged to 1.6.2.1. |
||||
|
||||
* "git fast-export" produced wrong output with some parents missing from |
||||
commits, when the history is clock-skewed. |
||||
|
||||
* "git fast-import" sometimes failed to read back objects it just wrote |
||||
out and aborted, because it failed to flush stale cached data. |
||||
|
||||
* "git-ls-tree" and "git-diff-tree" used a pathspec correctly when |
||||
deciding to descend into a subdirectory but they did not match the |
||||
individual paths correctly. This caused pathspecs "abc/d ab" to match |
||||
"abc/0" ("abc/d" made them decide to descend into the directory "abc/", |
||||
and then "ab" incorrectly matched "abc/0" when it shouldn't). |
||||
This fix was first merged to 1.6.2.3. |
||||
|
||||
* import-zips script (in contrib) did not compute the common directory |
||||
prefix correctly. |
||||
This fix was first merged to 1.6.2.2. |
||||
|
||||
* "git init" segfaulted when given an overlong template location via |
||||
the --template= option. |
||||
This fix was first merged to 1.6.2.4. |
||||
|
||||
* "git repack" did not error out when necessary object was missing in the |
||||
repository. |
||||
|
||||
* git-repack (invoked from git-gc) did not work as nicely as it should in |
||||
a repository that borrows objects from neighbours via alternates |
||||
mechanism especially when some packs are marked with the ".keep" flag |
||||
to prevent them from being repacked. |
||||
This fix was first merged to 1.6.2.3. |
||||
|
||||
Also includes minor documentation fixes and updates. |
||||
|
||||
-- |
||||
git shortlog --no-merges v1.6.1.3.. |
@ -0,0 +1,286 @@
@@ -0,0 +1,286 @@
|
||||
GIT v1.6.1 Release Notes |
||||
======================== |
||||
|
||||
Updates since v1.6.0 |
||||
-------------------- |
||||
|
||||
When some commands (e.g. "git log", "git diff") spawn pager internally, we |
||||
used to make the pager the parent process of the git command that produces |
||||
output. This meant that the exit status of the whole thing comes from the |
||||
pager, not the underlying git command. We swapped the order of the |
||||
processes around and you will see the exit code from the command from now |
||||
on. |
||||
|
||||
(subsystems) |
||||
|
||||
* gitk can call out to git-gui to view "git blame" output; git-gui in turn |
||||
can run gitk from its blame view. |
||||
|
||||
* Various git-gui updates including updated translations. |
||||
|
||||
* Various gitweb updates from repo.or.cz installation. |
||||
|
||||
* Updates to emacs bindings. |
||||
|
||||
(portability) |
||||
|
||||
* A few test scripts used nonportable "grep" that did not work well on |
||||
some platforms, e.g. Solaris. |
||||
|
||||
* Sample pre-auto-gc script has OS X support. |
||||
|
||||
* Makefile has support for (ancient) FreeBSD 4.9. |
||||
|
||||
(performance) |
||||
|
||||
* Many operations that are lstat(3) heavy can be told to pre-execute |
||||
necessary lstat(3) in parallel before their main operations, which |
||||
potentially gives much improved performance for cold-cache cases or in |
||||
environments with weak metadata caching (e.g. NFS). |
||||
|
||||
* The underlying diff machinery to produce textual output has been |
||||
optimized, which would result in faster "git blame" processing. |
||||
|
||||
* Most of the test scripts (but not the ones that try to run servers) |
||||
can be run in parallel. |
||||
|
||||
* Bash completion of refnames in a repository with massive number of |
||||
refs has been optimized. |
||||
|
||||
* Cygwin port uses native stat/lstat implementations when applicable, |
||||
which leads to improved performance. |
||||
|
||||
* "git push" pays attention to alternate repositories to avoid sending |
||||
unnecessary objects. |
||||
|
||||
* "git svn" can rebuild an out-of-date rev_map file. |
||||
|
||||
(usability, bells and whistles) |
||||
|
||||
* When you mistype a command name, git helpfully suggests what it guesses |
||||
you might have meant to say. help.autocorrect configuration can be set |
||||
to a non-zero value to accept the suggestion when git can uniquely |
||||
guess. |
||||
|
||||
* The packfile machinery hopefully is more robust when dealing with |
||||
corrupt packs if redundant objects involved in the corruption are |
||||
available elsewhere. |
||||
|
||||
* "git add -N path..." adds the named paths as an empty blob, so that |
||||
subsequent "git diff" will show a diff as if they are creation events. |
||||
|
||||
* "git add" gained a built-in synonym for people who want to say "stage |
||||
changes" instead of "add contents to the staging area" which amounts |
||||
to the same thing. |
||||
|
||||
* "git apply" learned --include=paths option, similar to the existing |
||||
--exclude=paths option. |
||||
|
||||
* "git bisect" is careful about a user mistake and suggests testing of |
||||
merge base first when good is not a strict ancestor of bad. |
||||
|
||||
* "git bisect skip" can take a range of commits. |
||||
|
||||
* "git blame" re-encodes the commit metainfo to UTF-8 from i18n.commitEncoding |
||||
by default. |
||||
|
||||
* "git check-attr --stdin" can check attributes for multiple paths. |
||||
|
||||
* "git checkout --track origin/hack" used to be a syntax error. It now |
||||
DWIMs to create a corresponding local branch "hack", i.e. acts as if you |
||||
said "git checkout --track -b hack origin/hack". |
||||
|
||||
* "git checkout --ours/--theirs" can be used to check out one side of a |
||||
conflicting merge during conflict resolution. |
||||
|
||||
* "git checkout -m" can be used to recreate the initial conflicted state |
||||
during conflict resolution. |
||||
|
||||
* "git cherry-pick" can also utilize rerere for conflict resolution. |
||||
|
||||
* "git clone" learned to be verbose with -v |
||||
|
||||
* "git commit --author=$name" can look up author name from existing |
||||
commits. |
||||
|
||||
* output from "git commit" has been reworded in a more concise and yet |
||||
more informative way. |
||||
|
||||
* "git count-objects" reports the on-disk footprint for packfiles and |
||||
their corresponding idx files. |
||||
|
||||
* "git daemon" learned --max-connections=<count> option. |
||||
|
||||
* "git daemon" exports REMOTE_ADDR to record client address, so that |
||||
spawned programs can act differently on it. |
||||
|
||||
* "git describe --tags" favours closer lightweight tags than farther |
||||
annotated tags now. |
||||
|
||||
* "git diff" learned to mimic --suppress-blank-empty from GNU diff via a |
||||
configuration option. |
||||
|
||||
* "git diff" learned to put more sensible hunk headers for Python, |
||||
HTML and ObjC contents. |
||||
|
||||
* "git diff" learned to vary the a/ vs b/ prefix depending on what are |
||||
being compared, controlled by diff.mnemonicprefix configuration. |
||||
|
||||
* "git diff" learned --dirstat-by-file to count changed files, not number |
||||
of lines, when summarizing the global picture. |
||||
|
||||
* "git diff" learned "textconv" filters --- a binary or hard-to-read |
||||
contents can be munged into human readable form and the difference |
||||
between the results of the conversion can be viewed (obviously this |
||||
cannot produce a patch that can be applied, so this is disabled in |
||||
format-patch among other things). |
||||
|
||||
* "--cached" option to "git diff has an easier to remember synonym "--staged", |
||||
to ask "what is the difference between the given commit and the |
||||
contents staged in the index?" |
||||
|
||||
* "git for-each-ref" learned "refname:short" token that gives an |
||||
unambiguously abbreviated refname. |
||||
|
||||
* Auto-numbering of the subject lines is the default for "git |
||||
format-patch" now. |
||||
|
||||
* "git grep" learned to accept -z similar to GNU grep. |
||||
|
||||
* "git help" learned to use GIT_MAN_VIEWER environment variable before |
||||
using "man" program. |
||||
|
||||
* "git imap-send" can optionally talk SSL. |
||||
|
||||
* "git index-pack" is more careful against disk corruption while |
||||
completing a thin pack. |
||||
|
||||
* "git log --check" and "git log --exit-code" passes their underlying diff |
||||
status with their exit status code. |
||||
|
||||
* "git log" learned --simplify-merges, a milder variant of --full-history; |
||||
"gitk --simplify-merges" is easier to view than with --full-history. |
||||
|
||||
* "git log" learned "--source" to show what ref each commit was reached |
||||
from. |
||||
|
||||
* "git log" also learned "--simplify-by-decoration" to show the |
||||
birds-eye-view of the topology of the history. |
||||
|
||||
* "git log --pretty=format:" learned "%d" format element that inserts |
||||
names of tags that point at the commit. |
||||
|
||||
* "git merge --squash" and "git merge --no-ff" into an unborn branch are |
||||
noticed as user errors. |
||||
|
||||
* "git merge -s $strategy" can use a custom built strategy if you have a |
||||
command "git-merge-$strategy" on your $PATH. |
||||
|
||||
* "git pull" (and "git fetch") can be told to operate "-v"erbosely or |
||||
"-q"uietly. |
||||
|
||||
* "git push" can be told to reject deletion of refs with receive.denyDeletes |
||||
configuration. |
||||
|
||||
* "git rebase" honours pre-rebase hook; use --no-verify to bypass it. |
||||
|
||||
* "git rebase -p" uses interactive rebase machinery now to preserve the merges. |
||||
|
||||
* "git reflog expire branch" can be used in place of "git reflog expire |
||||
refs/heads/branch". |
||||
|
||||
* "git remote show $remote" lists remote branches one-per-line now. |
||||
|
||||
* "git send-email" can be given revision range instead of files and |
||||
maildirs on the command line, and automatically runs format-patch to |
||||
generate patches for the given revision range. |
||||
|
||||
* "git submodule foreach" subcommand allows you to iterate over checked |
||||
out submodules. |
||||
|
||||
* "git submodule sync" subcommands allows you to update the origin URL |
||||
recorded in submodule directories from the toplevel .gitmodules file. |
||||
|
||||
* "git svn branch" can create new branches on the other end. |
||||
|
||||
* "gitweb" can use more saner PATH_INFO based URL. |
||||
|
||||
(internal) |
||||
|
||||
* "git hash-object" learned to lie about the path being hashed, so that |
||||
correct gitattributes processing can be done while hashing contents |
||||
stored in a temporary file. |
||||
|
||||
* various callers of git-merge-recursive avoid forking it as an external |
||||
process. |
||||
|
||||
* Git class defined in "Git.pm" can be subclasses a bit more easily. |
||||
|
||||
* We used to link GNU regex library as a compatibility layer for some |
||||
platforms, but it turns out it is not necessary on most of them. |
||||
|
||||
* Some path handling routines used fixed number of buffers used alternately |
||||
but depending on the call depth, this arrangement led to hard to track |
||||
bugs. This issue is being addressed. |
||||
|
||||
|
||||
Fixes since v1.6.0 |
||||
------------------ |
||||
|
||||
All of the fixes in v1.6.0.X maintenance series are included in this |
||||
release, unless otherwise noted. |
||||
|
||||
* Porcelains implemented as shell scripts were utterly confused when you |
||||
entered to a subdirectory of a work tree from sideways, following a |
||||
symbolic link (this may need to be backported to older releases later). |
||||
|
||||
* Tracking symbolic links would work better on filesystems whose lstat() |
||||
returns incorrect st_size value for them. |
||||
|
||||
* "git add" and "git update-index" incorrectly allowed adding S/F when S |
||||
is a tracked symlink that points at a directory D that has a path F in |
||||
it (we still need to fix a similar nonsense when S is a submodule and F |
||||
is a path in it). |
||||
|
||||
* "git am" after stopping at a broken patch lost --whitespace, -C, -p and |
||||
--3way options given from the command line initially. |
||||
|
||||
* "git diff --stdin" used to take two trees on a line and compared them, |
||||
but we dropped support for such a use case long time ago. This has |
||||
been resurrected. |
||||
|
||||
* "git filter-branch" failed to rewrite a tag name with slashes in it. |
||||
|
||||
* "git http-push" did not understand URI scheme other than opaquelocktoken |
||||
when acquiring a lock from the server (this may need to be backported to |
||||
older releases later). |
||||
|
||||
* After "git rebase -p" stopped with conflicts while replaying a merge, |
||||
"git rebase --continue" did not work (may need to be backported to older |
||||
releases). |
||||
|
||||
* "git revert" records relative to which parent a revert was made when |
||||
reverting a merge. Together with new documentation that explains issues |
||||
around reverting a merge and merging from the updated branch later, this |
||||
hopefully will reduce user confusion (this may need to be backported to |
||||
older releases later). |
||||
|
||||
* "git rm --cached" used to allow an empty blob that was added earlier to |
||||
be removed without --force, even when the file in the work tree has |
||||
since been modified. |
||||
|
||||
* "git push --tags --all $there" failed with generic usage message without |
||||
telling saying these two options are incompatible. |
||||
|
||||
* "git log --author/--committer" match used to potentially match the |
||||
timestamp part, exposing internal implementation detail. Also these did |
||||
not work with --fixed-strings match at all. |
||||
|
||||
* "gitweb" did not mark non-ASCII characters imported from external HTML fragments |
||||
correctly. |
||||
|
||||
-- |
||||
exec >/var/tmp/1 |
||||
O=v1.6.1-rc3-74-gf66bc5f |
||||
echo O=$(git describe master) |
||||
git shortlog --no-merges $O..master ^maint |
@ -0,0 +1,19 @@
@@ -0,0 +1,19 @@
|
||||
GIT v1.6.2.1 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.2 |
||||
------------------ |
||||
|
||||
* .gitignore learned to handle backslash as a quoting mechanism for |
||||
comment introduction character "#". |
||||
|
||||
* timestamp output in --date=relative mode used to display timestamps that |
||||
are long time ago in the default mode; it now uses "N years M months |
||||
ago", and "N years ago". |
||||
|
||||
* git-add -i/-p now works with non-ASCII pathnames. |
||||
|
||||
* "git hash-object -w" did not read from the configuration file from the |
||||
correct .git directory. |
||||
|
||||
* git-send-email learned to correctly handle multiple Cc: addresses. |
@ -0,0 +1,45 @@
@@ -0,0 +1,45 @@
|
||||
GIT v1.6.2.2 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.2.1 |
||||
-------------------- |
||||
|
||||
* A longstanding confusing description of what --pickaxe option of |
||||
git-diff does has been clarified in the documentation. |
||||
|
||||
* "git-blame -S" did not quite work near the commits that were given |
||||
on the command line correctly. |
||||
|
||||
* "git diff --pickaxe-regexp" did not count overlapping matches |
||||
correctly. |
||||
|
||||
* "git diff" did not feed files in work-tree representation to external |
||||
diff and textconv. |
||||
|
||||
* "git-fetch" in a repository that was not cloned from anywhere said |
||||
it cannot find 'origin', which was hard to understand for new people. |
||||
|
||||
* "git-format-patch --numbered-files --stdout" did not have to die of |
||||
incompatible options; it now simply ignores --numbered-files as no files |
||||
are produced anyway. |
||||
|
||||
* "git-ls-files --deleted" did not work well with GIT_DIR&GIT_WORK_TREE. |
||||
|
||||
* "git-read-tree A B C..." without -m option has been broken for a long |
||||
time. |
||||
|
||||
* git-send-email ignored --in-reply-to when --no-thread was given. |
||||
|
||||
* 'git-submodule add' did not tolerate extra slashes and ./ in the path it |
||||
accepted from the command line; it now is more lenient. |
||||
|
||||
* git-svn misbehaved when the project contained a path that began with |
||||
two dashes. |
||||
|
||||
* import-zips script (in contrib) did not compute the common directory |
||||
prefix correctly. |
||||
|
||||
* miscompilation of negated enum constants by old gcc (2.9) affected the |
||||
codepaths to spawn subprocesses. |
||||
|
||||
Many small documentation updates are included as well. |
@ -0,0 +1,22 @@
@@ -0,0 +1,22 @@
|
||||
GIT v1.6.2.3 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.2.2 |
||||
-------------------- |
||||
|
||||
* Setting an octal mode value to core.sharedrepository configuration to |
||||
restrict access to the repository to group members did not work as |
||||
advertised. |
||||
|
||||
* A fairly large and trivial memory leak while rev-list shows list of |
||||
reachable objects has been identified and plugged. |
||||
|
||||
* "git-commit --interactive" did not abort when underlying "git-add -i" |
||||
signaled a failure. |
||||
|
||||
* git-repack (invoked from git-gc) did not work as nicely as it should in |
||||
a repository that borrows objects from neighbours via alternates |
||||
mechanism especially when some packs are marked with the ".keep" flag |
||||
to prevent them from being repacked. |
||||
|
||||
Many small documentation updates are included as well. |
@ -0,0 +1,39 @@
@@ -0,0 +1,39 @@
|
||||
GIT v1.6.2.4 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.2.3 |
||||
-------------------- |
||||
|
||||
* The configuration parser had a buffer overflow while parsing an overlong |
||||
value. |
||||
|
||||
* pruning reflog entries that are unreachable from the tip of the ref |
||||
during "git reflog prune" (hence "git gc") was very inefficient. |
||||
|
||||
* "git-add -p" lacked a way to say "q"uit to refuse staging any hunks for |
||||
the remaining paths. You had to say "d" and then ^C. |
||||
|
||||
* "git-checkout <tree-ish> <submodule>" did not update the index entry at |
||||
the named path; it now does. |
||||
|
||||
* "git-fast-export" choked when seeing a tag that does not point at commit. |
||||
|
||||
* "git init" segfaulted when given an overlong template location via |
||||
the --template= option. |
||||
|
||||
* "git-ls-tree" and "git-diff-tree" used a pathspec correctly when |
||||
deciding to descend into a subdirectory but they did not match the |
||||
individual paths correctly. This caused pathspecs "abc/d ab" to match |
||||
"abc/0" ("abc/d" made them decide to descend into the directory "abc/", |
||||
and then "ab" incorrectly matched "abc/0" when it shouldn't). |
||||
|
||||
* "git-merge-recursive" was broken when a submodule entry was involved in |
||||
a criss-cross merge situation. |
||||
|
||||
Many small documentation updates are included as well. |
||||
|
||||
--- |
||||
exec >/var/tmp/1 |
||||
echo O=$(git describe maint) |
||||
O=v1.6.2.3-38-g318b847 |
||||
git shortlog --no-merges $O..maint |
@ -0,0 +1,21 @@
@@ -0,0 +1,21 @@
|
||||
GIT v1.6.2.5 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.2.4 |
||||
-------------------- |
||||
|
||||
* "git apply" mishandled if you fed a git generated patch that renames |
||||
file A to B and file B to A at the same time. |
||||
|
||||
* "git diff -c -p" (and "diff --cc") did not expect to see submodule |
||||
differences and instead refused to work. |
||||
|
||||
* "git grep -e '('" segfaulted, instead of diagnosing a mismatched |
||||
parentheses error. |
||||
|
||||
* "git fetch" generated packs with offset-delta encoding when both ends of |
||||
the connection are capable of producing one; this cannot be read by |
||||
ancient git and the user should be able to disable this by setting |
||||
repack.usedeltabaseoffset configuration to false. |
||||
|
||||
|
@ -0,0 +1,164 @@
@@ -0,0 +1,164 @@
|
||||
GIT v1.6.2 Release Notes |
||||
======================== |
||||
|
||||
With the next major release, "git push" into a branch that is |
||||
currently checked out will be refused by default. You can choose |
||||
what should happen upon such a push by setting the configuration |
||||
variable receive.denyCurrentBranch in the receiving repository. |
||||
|
||||
To ease the transition plan, the receiving repository of such a |
||||
push running this release will issue a big warning when the |
||||
configuration variable is missing. Please refer to: |
||||
|
||||
http://git.or.cz/gitwiki/GitFaq#non-bare |
||||
http://thread.gmane.org/gmane.comp.version-control.git/107758/focus=108007 |
||||
|
||||
for more details on the reason why this change is needed and the |
||||
transition plan. |
||||
|
||||
For a similar reason, "git push $there :$killed" to delete the branch |
||||
$killed in a remote repository $there, if $killed branch is the current |
||||
branch pointed at by its HEAD, gets a large warning. You can choose what |
||||
should happen upon such a push by setting the configuration variable |
||||
receive.denyDeleteCurrent in the receiving repository. |
||||
|
||||
|
||||
Updates since v1.6.1 |
||||
-------------------- |
||||
|
||||
(subsystems) |
||||
|
||||
* git-svn updates. |
||||
|
||||
* gitweb updates, including a new patch view and RSS/Atom feed |
||||
improvements. |
||||
|
||||
* (contrib/emacs) git.el now has commands for checking out a branch, |
||||
creating a branch, cherry-picking and reverting commits; vc-git.el |
||||
is not shipped with git anymore (it is part of official Emacs). |
||||
|
||||
(performance) |
||||
|
||||
* pack-objects autodetects the number of CPUs available and uses threaded |
||||
version. |
||||
|
||||
(usability, bells and whistles) |
||||
|
||||
* automatic typo correction works on aliases as well |
||||
|
||||
* @{-1} is a way to refer to the last branch you were on. This is |
||||
accepted not only where an object name is expected, but anywhere |
||||
a branch name is expected and acts as if you typed the branch name. |
||||
E.g. "git branch --track mybranch @{-1}", "git merge @{-1}", and |
||||
"git rev-parse --symbolic-full-name @{-1}" would work as expected. |
||||
|
||||
* When refs/remotes/origin/HEAD points at a remote tracking branch that |
||||
has been pruned away, many git operations issued warning when they |
||||
internally enumerated the refs. We now warn only when you say "origin" |
||||
to refer to that pruned branch. |
||||
|
||||
* The location of .mailmap file can be configured, and its file format was |
||||
enhanced to allow mapping an incorrect e-mail field as well. |
||||
|
||||
* "git add -p" learned 'g'oto action to jump directly to a hunk. |
||||
|
||||
* "git add -p" learned to find a hunk with given text with '/'. |
||||
|
||||
* "git add -p" optionally can be told to work with just the command letter |
||||
without Enter. |
||||
|
||||
* when "git am" stops upon a patch that does not apply, it shows the |
||||
title of the offending patch. |
||||
|
||||
* "git am --directory=<dir>" and "git am --reject" passes these options |
||||
to underlying "git apply". |
||||
|
||||
* "git am" learned --ignore-date option. |
||||
|
||||
* "git blame" aligns author names better when they are spelled in |
||||
non US-ASCII encoding. |
||||
|
||||
* "git clone" now makes its best effort when cloning from an empty |
||||
repository to set up configuration variables to refer to the remote |
||||
repository. |
||||
|
||||
* "git checkout -" is a shorthand for "git checkout @{-1}". |
||||
|
||||
* "git cherry" defaults to whatever the current branch is tracking (if |
||||
exists) when the <upstream> argument is not given. |
||||
|
||||
* "git cvsserver" can be told not to add extra "via git-CVS emulator" to |
||||
the commit log message it serves via gitcvs.commitmsgannotation |
||||
configuration. |
||||
|
||||
* "git cvsserver" learned to handle 'noop' command some CVS clients seem |
||||
to expect to work. |
||||
|
||||
* "git diff" learned a new option --inter-hunk-context to coalesce close |
||||
hunks together and show context between them. |
||||
|
||||
* The definition of what constitutes a word for "git diff --color-words" |
||||
can be customized via gitattributes, command line or a configuration. |
||||
|
||||
* "git diff" learned --patience to run "patience diff" algorithm. |
||||
|
||||
* "git filter-branch" learned --prune-empty option that discards commits |
||||
that do not change the contents. |
||||
|
||||
* "git fsck" now checks loose objects in alternate object stores, instead |
||||
of misreporting them as missing. |
||||
|
||||
* "git gc --prune" was resurrected to allow "git gc --no-prune" and |
||||
giving non-default expiration period e.g. "git gc --prune=now". |
||||
|
||||
* "git grep -w" and "git grep" for fixed strings have been optimized. |
||||
|
||||
* "git mergetool" learned -y(--no-prompt) option to disable prompting. |
||||
|
||||
* "git rebase -i" can transplant a history down to root to elsewhere |
||||
with --root option. |
||||
|
||||
* "git reset --merge" is a new mode that works similar to the way |
||||
"git checkout" switches branches, taking the local changes while |
||||
switching to another commit. |
||||
|
||||
* "git submodule update" learned --no-fetch option. |
||||
|
||||
* "git tag" learned --contains that works the same way as the same option |
||||
from "git branch". |
||||
|
||||
|
||||
Fixes since v1.6.1 |
||||
------------------ |
||||
|
||||
All of the fixes in v1.6.1.X maintenance series are included in this |
||||
release, unless otherwise noted. |
||||
|
||||
Here are fixes that this release has, but have not been backported to |
||||
v1.6.1.X series. |
||||
|
||||
* "git-add sub/file" when sub is a submodule incorrectly added the path to |
||||
the superproject. |
||||
|
||||
* "git bundle" did not exclude annotated tags even when a range given |
||||
from the command line wanted to. |
||||
|
||||
* "git filter-branch" unnecessarily refused to work when you had |
||||
checked out a different commit from what is recorded in the superproject |
||||
index in a submodule. |
||||
|
||||
* "git filter-branch" incorrectly tried to update a nonexistent work tree |
||||
at the end when it is run in a bare repository. |
||||
|
||||
* "git gc" did not work if your repository was created with an ancient git |
||||
and never had any pack files in it before. |
||||
|
||||
* "git mergetool" used to ignore autocrlf and other attributes |
||||
based content rewriting. |
||||
|
||||
* branch switching and merges had a silly bug that did not validate |
||||
the correct directory when making sure an existing subdirectory is |
||||
clean. |
||||
|
||||
* "git -p cmd" when cmd is not a built-in one left the display in funny state |
||||
when killed in the middle. |
@ -0,0 +1,12 @@
@@ -0,0 +1,12 @@
|
||||
GIT v1.6.3.1 Release Notes |
||||
========================== |
||||
|
||||
Fixes since v1.6.3 |
||||
------------------ |
||||
|
||||
-- |
||||
exec >/var/tmp/1 |
||||
O=v1.6.3 |
||||
echo O=$(git describe maint) |
||||
git shortlog $O..maint |
||||
|
@ -0,0 +1,182 @@
@@ -0,0 +1,182 @@
|
||||
GIT v1.6.3 Release Notes |
||||
======================== |
||||
|
||||
With the next major release, "git push" into a branch that is |
||||
currently checked out will be refused by default. You can choose |
||||
what should happen upon such a push by setting the configuration |
||||
variable receive.denyCurrentBranch in the receiving repository. |
||||
|
||||
To ease the transition plan, the receiving repository of such a |
||||
push running this release will issue a big warning when the |
||||
configuration variable is missing. Please refer to: |
||||
|
||||
http://git.or.cz/gitwiki/GitFaq#non-bare |
||||
http://thread.gmane.org/gmane.comp.version-control.git/107758/focus=108007 |
||||
|
||||
for more details on the reason why this change is needed and the |
||||
transition plan. |
||||
|
||||
For a similar reason, "git push $there :$killed" to delete the branch |
||||
$killed in a remote repository $there, if $killed branch is the current |
||||
branch pointed at by its HEAD, gets a large warning. You can choose what |
||||
should happen upon such a push by setting the configuration variable |
||||
receive.denyDeleteCurrent in the receiving repository. |
||||
|
||||
When the user does not tell "git push" what to push, it has always |
||||
pushed matching refs. For some people it is unexpected, and a new |
||||
configuration variable push.default has been introduced to allow |
||||
changing a different default behaviour. To advertise the new feature, |
||||
a big warning is issued if this is not configured and a git push without |
||||
arguments is attempted. |
||||
|
||||
|
||||
Updates since v1.6.2 |
||||
-------------------- |
||||
|
||||
(subsystems) |
||||
|
||||
* various git-svn updates. |
||||
|
||||
* git-gui updates, including an update to Russian translation, and a |
||||
fix to an infinite loop when showing an empty diff. |
||||
|
||||
* gitk updates, including an update to Russian translation and improved Windows |
||||
support. |
||||
|
||||
(performance) |
||||
|
||||
* many uses of lstat(2) in the codepath for "git checkout" have been |
||||
optimized out. |
||||
|
||||
(usability, bells and whistles) |
||||
|
||||
* Boolean configuration variable yes/no can be written as on/off. |
||||
|
||||
* rsync:/path/to/repo can be used to run git over rsync for local |
||||
repositories. It may not be useful in practice; meant primarily for |
||||
testing. |
||||
|
||||
* http transport learned to prompt and use password when fetching from or |
||||
pushing to http://user@host.xz/ URL. |
||||
|
||||
* (msysgit) progress output that is sent over the sideband protocol can |
||||
be handled appropriately in Windows console. |
||||
|
||||
* "--pretty=<style>" option to the log family of commands can now be |
||||
spelled as "--format=<style>". In addition, --format=%formatstring |
||||
is a short-hand for --pretty=tformat:%formatstring. |
||||
|
||||
* "--oneline" is a synonym for "--pretty=oneline --abbrev-commit". |
||||
|
||||
* "--graph" to the "git log" family can draw the commit ancestry graph |
||||
in colors. |
||||
|
||||
* If you realize that you botched the patch when you are editing hunks |
||||
with the 'edit' action in git-add -i/-p, you can abort the editor to |
||||
tell git not to apply it. |
||||
|
||||
* @{-1} is a new way to refer to the last branch you were on introduced in |
||||
1.6.2, but the initial implementation did not teach this to a few |
||||
commands. Now the syntax works with "branch -m @{-1} newname". |
||||
|
||||
* git-archive learned --output=<file> option. |
||||
|
||||
* git-archive takes attributes from the tree being archived; strictly |
||||
speaking, this is an incompatible behaviour change, but is a good one. |
||||
Use --worktree-attributes option to allow it to read attributes from |
||||
the work tree as before (deprecated git-tar tree command always reads |
||||
attributes from the work tree). |
||||
|
||||
* git-bisect shows not just the number of remaining commits whose goodness |
||||
is unknown, but also shows the estimated number of remaining rounds. |
||||
|
||||
* You can give --date=<format> option to git-blame. |
||||
|
||||
* "git-branch -r" shows HEAD symref that points at a remote branch in |
||||
interest of each tracked remote repository. |
||||
|
||||
* "git-branch -v -v" is a new way to get list of names for branches and the |
||||
"upstream" branch for them. |
||||
|
||||
* git-config learned -e option to open an editor to edit the config file |
||||
directly. |
||||
|
||||
* git-clone runs post-checkout hook when run without --no-checkout. |
||||
|
||||
* git-difftool is now part of the officially supported command, primarily |
||||
maintained by David Aguilar. |
||||
|
||||
* git-for-each-ref learned a new "upstream" token. |
||||
|
||||
* git-format-patch can be told to use attachment with a new configuration, |
||||
format.attach. |
||||
|
||||
* git-format-patch can be told to produce deep or shallow message threads. |
||||
|
||||
* git-format-patch can be told to always add sign-off with a configuration |
||||
variable. |
||||
|
||||
* git-format-patch learned format.headers configuration to add extra |
||||
header fields to the output. This behaviour is similar to the existing |
||||
--add-header=<header> option of the command. |
||||
|
||||
* git-format-patch gives human readable names to the attached files, when |
||||
told to send patches as attachments. |
||||
|
||||
* git-grep learned to highlight the found substrings in color. |
||||
|
||||
* git-imap-send learned to work around Thunderbird's inability to easily |
||||
disable format=flowed with a new configuration, imap.preformattedHTML. |
||||
|
||||
* git-rebase can be told to rebase the series even if your branch is a |
||||
descendant of the commit you are rebasing onto with --force-rebase |
||||
option. |
||||
|
||||
* git-rebase can be told to report diffstat with the --stat option. |
||||
|
||||
* Output from git-remote command has been vastly improved. |
||||
|
||||
* "git remote update --prune $remote" updates from the named remote and |
||||
then prunes stale tracking branches. |
||||
|
||||
* git-send-email learned --confirm option to review the Cc: list before |
||||
sending the messages out. |
||||
|
||||
(developers) |
||||
|
||||
* Test scripts can be run under valgrind. |
||||
|
||||
* Test scripts can be run with installed git. |
||||
|
||||
* Makefile learned 'coverage' option to run the test suites with |
||||
coverage tracking enabled. |
||||
|
||||
* Building the manpages with docbook-xsl between 1.69.1 and 1.71.1 now |
||||
requires setting DOCBOOK_SUPPRESS_SP to work around a docbook-xsl bug. |
||||
This workaround used to be enabled by default, but causes problems |
||||
with newer versions of docbook-xsl. In addition, there are a few more |
||||
knobs you can tweak to work around issues with various versions of the |
||||
docbook-xsl package. See comments in Documentation/Makefile for details. |
||||
|
||||
* Support for building and testing a subset of git on a system without a |
||||
working perl has been improved. |
||||
|
||||
|
||||
Fixes since v1.6.2 |
||||
------------------ |
||||
|
||||
All of the fixes in v1.6.2.X maintenance series are included in this |
||||
release, unless otherwise noted. |
||||
|
||||
Here are fixes that this release has, but have not been backported to |
||||
v1.6.2.X series. |
||||
|
||||
* "git-apply" rejected a patch that swaps two files (i.e. renames A to B |
||||
and B to A at the same time). May need to be backported by cherry |
||||
picking d8c81df and then 7fac0ee). |
||||
|
||||
* The initial checkout did not read the attributes from the .gitattribute |
||||
file that is being checked out. |
||||
|
||||
* git-gc spent excessive amount of time to decide if an object appears |
||||
in a locally existing pack (if needed, backport by merging 69e020a). |
@ -0,0 +1,59 @@
@@ -0,0 +1,59 @@
|
||||
GIT v1.6.4 Release Notes |
||||
======================== |
||||
|
||||
With the next major release, "git push" into a branch that is |
||||
currently checked out will be refused by default. You can choose |
||||
what should happen upon such a push by setting the configuration |
||||
variable receive.denyCurrentBranch in the receiving repository. |
||||
|
||||
To ease the transition plan, the receiving repository of such a |
||||
push running this release will issue a big warning when the |
||||
configuration variable is missing. Please refer to: |
||||
|
||||
http://git.or.cz/gitwiki/GitFaq#non-bare |
||||
http://thread.gmane.org/gmane.comp.version-control.git/107758/focus=108007 |
||||
|
||||
for more details on the reason why this change is needed and the |
||||
transition plan. |
||||
|
||||
For a similar reason, "git push $there :$killed" to delete the branch |
||||
$killed in a remote repository $there, if $killed branch is the current |
||||
branch pointed at by its HEAD, gets a large warning. You can choose what |
||||
should happen upon such a push by setting the configuration variable |
||||
receive.denyDeleteCurrent in the receiving repository. |
||||
|
||||
When the user does not tell "git push" what to push, it has always |
||||
pushed matching refs. For some people it is unexpected, and a new |
||||
configuration variable push.default has been introduced to allow |
||||
changing a different default behaviour. To advertise the new feature, |
||||
a big warning is issued if this is not configured and a git push without |
||||
arguments is attempted. |
||||
|
||||
|
||||
Updates since v1.6.3 |
||||
-------------------- |
||||
|
||||
(subsystems) |
||||
|
||||
(performance) |
||||
|
||||
(usability, bells and whistles) |
||||
|
||||
(developers) |
||||
|
||||
|
||||
Fixes since v1.6.3 |
||||
------------------ |
||||
|
||||
All of the fixes in v1.6.3.X maintenance series are included in this |
||||
release, unless otherwise noted. |
||||
|
||||
Here are fixes that this release has, but have not been backported to |
||||
v1.6.3.X series. |
||||
|
||||
|
||||
--- |
||||
exec >/var/tmp/1 |
||||
echo O=$(git describe master) |
||||
O=v1.6.3 |
||||
git shortlog --no-merges $O..master ^maint |
@ -1,30 +0,0 @@
@@ -1,30 +0,0 @@
|
||||
<!-- callout.xsl: converts asciidoc callouts to man page format --> |
||||
<xsl:stylesheet xmlns:xsl="http://www.w3.org/1999/XSL/Transform" version="1.0"> |
||||
<xsl:template match="co"> |
||||
<xsl:value-of select="concat('\fB(',substring-after(@id,'-'),')\fR')"/> |
||||
</xsl:template> |
||||
<xsl:template match="calloutlist"> |
||||
<xsl:text>.sp </xsl:text> |
||||
<xsl:apply-templates/> |
||||
<xsl:text> </xsl:text> |
||||
</xsl:template> |
||||
<xsl:template match="callout"> |
||||
<xsl:value-of select="concat('\fB',substring-after(@arearefs,'-'),'. \fR')"/> |
||||
<xsl:apply-templates/> |
||||
<xsl:text>.br </xsl:text> |
||||
</xsl:template> |
||||
|
||||
<!-- sorry, this is not about callouts, but attempts to work around |
||||
spurious .sp at the tail of the line docbook stylesheets seem to add --> |
||||
<xsl:template match="simpara"> |
||||
<xsl:variable name="content"> |
||||
<xsl:apply-templates/> |
||||
</xsl:variable> |
||||
<xsl:value-of select="normalize-space($content)"/> |
||||
<xsl:if test="not(ancestor::authorblurb) and |
||||
not(ancestor::personblurb)"> |
||||
<xsl:text> </xsl:text> |
||||
</xsl:if> |
||||
</xsl:template> |
||||
|
||||
</xsl:stylesheet> |
@ -0,0 +1,42 @@
@@ -0,0 +1,42 @@
|
||||
#!/usr/bin/perl -w |
||||
|
||||
my @menu = (); |
||||
my $output = $ARGV[0]; |
||||
|
||||
open TMP, '>', "$output.tmp"; |
||||
|
||||
while (<STDIN>) { |
||||
next if (/^\\input texinfo/../\@node Top/); |
||||
next if (/^\@bye/ || /^\.ft/); |
||||
if (s/^\@top (.*)/\@node $1,,,Top/) { |
||||
push @menu, $1; |
||||
} |
||||
s/\(\@pxref{\[(URLS|REMOTES)\]}\)//; |
||||
print TMP; |
||||
} |
||||
close TMP; |
||||
|
||||
printf '\input texinfo |
||||
@setfilename gitman.info |
||||
@documentencoding UTF-8 |
||||
@dircategory Development |
||||
@direntry |
||||
* Git Man Pages: (gitman). Manual pages for Git revision control system |
||||
@end direntry |
||||
@node Top,,, (dir) |
||||
@top Git Manual Pages |
||||
@documentlanguage en |
||||
@menu |
||||
', $menu[0]; |
||||
|
||||
for (@menu) { |
||||
print "* ${_}::\n"; |
||||
} |
||||
print "\@end menu\n"; |
||||
open TMP, '<', "$output.tmp"; |
||||
while (<TMP>) { |
||||
print; |
||||
} |
||||
close TMP; |
||||
print "\@bye\n"; |
||||
unlink "$output.tmp"; |
@ -1,592 +0,0 @@
@@ -1,592 +0,0 @@
|
||||
//////////////////////////////////////////////////////////////// |
||||
|
||||
GIT - the stupid content tracker |
||||
|
||||
//////////////////////////////////////////////////////////////// |
||||
|
||||
"git" can mean anything, depending on your mood. |
||||
|
||||
- random three-letter combination that is pronounceable, and not |
||||
actually used by any common UNIX command. The fact that it is a |
||||
mispronunciation of "get" may or may not be relevant. |
||||
- stupid. contemptible and despicable. simple. Take your pick from the |
||||
dictionary of slang. |
||||
- "global information tracker": you're in a good mood, and it actually |
||||
works for you. Angels sing, and a light suddenly fills the room. |
||||
- "goddamn idiotic truckload of sh*t": when it breaks |
||||
|
||||
This is a (not so) stupid but extremely fast directory content manager. |
||||
It doesn't do a whole lot at its core, but what it 'does' do is track |
||||
directory contents efficiently. |
||||
|
||||
There are two object abstractions: the "object database", and the |
||||
"current directory cache" aka "index". |
||||
|
||||
The Object Database |
||||
~~~~~~~~~~~~~~~~~~~ |
||||
The object database is literally just a content-addressable collection |
||||
of objects. All objects are named by their content, which is |
||||
approximated by the SHA1 hash of the object itself. Objects may refer |
||||
to other objects (by referencing their SHA1 hash), and so you can |
||||
build up a hierarchy of objects. |
||||
|
||||
All objects have a statically determined "type" aka "tag", which is |
||||
determined at object creation time, and which identifies the format of |
||||
the object (i.e. how it is used, and how it can refer to other |
||||
objects). There are currently four different object types: "blob", |
||||
"tree", "commit" and "tag". |
||||
|
||||
A "blob" object cannot refer to any other object, and is, like the type |
||||
implies, a pure storage object containing some user data. It is used to |
||||
actually store the file data, i.e. a blob object is associated with some |
||||
particular version of some file. |
||||
|
||||
A "tree" object is an object that ties one or more "blob" objects into a |
||||
directory structure. In addition, a tree object can refer to other tree |
||||
objects, thus creating a directory hierarchy. |
||||
|
||||
A "commit" object ties such directory hierarchies together into |
||||
a DAG of revisions - each "commit" is associated with exactly one tree |
||||
(the directory hierarchy at the time of the commit). In addition, a |
||||
"commit" refers to one or more "parent" commit objects that describe the |
||||
history of how we arrived at that directory hierarchy. |
||||
|
||||
As a special case, a commit object with no parents is called the "root" |
||||
object, and is the point of an initial project commit. Each project |
||||
must have at least one root, and while you can tie several different |
||||
root objects together into one project by creating a commit object which |
||||
has two or more separate roots as its ultimate parents, that's probably |
||||
just going to confuse people. So aim for the notion of "one root object |
||||
per project", even if git itself does not enforce that. |
||||
|
||||
A "tag" object symbolically identifies and can be used to sign other |
||||
objects. It contains the identifier and type of another object, a |
||||
symbolic name (of course!) and, optionally, a signature. |
||||
|
||||
Regardless of object type, all objects share the following |
||||
characteristics: they are all deflated with zlib, and have a header |
||||
that not only specifies their type, but also provides size information |
||||
about the data in the object. It's worth noting that the SHA1 hash |
||||
that is used to name the object is the hash of the original data |
||||
plus this header, so `sha1sum` 'file' does not match the object name |
||||
for 'file'. |
||||
(Historical note: in the dawn of the age of git the hash |
||||
was the sha1 of the 'compressed' object.) |
||||
|
||||
As a result, the general consistency of an object can always be tested |
||||
independently of the contents or the type of the object: all objects can |
||||
be validated by verifying that (a) their hashes match the content of the |
||||
file and (b) the object successfully inflates to a stream of bytes that |
||||
forms a sequence of <ascii type without space> + <space> + <ascii decimal |
||||
size> + <byte\0> + <binary object data>. |
||||
|
||||
The structured objects can further have their structure and |
||||
connectivity to other objects verified. This is generally done with |
||||
the `git-fsck` program, which generates a full dependency graph |
||||
of all objects, and verifies their internal consistency (in addition |
||||
to just verifying their superficial consistency through the hash). |
||||
|
||||
The object types in some more detail: |
||||
|
||||
Blob Object |
||||
~~~~~~~~~~~ |
||||
A "blob" object is nothing but a binary blob of data, and doesn't |
||||
refer to anything else. There is no signature or any other |
||||
verification of the data, so while the object is consistent (it 'is' |
||||
indexed by its sha1 hash, so the data itself is certainly correct), it |
||||
has absolutely no other attributes. No name associations, no |
||||
permissions. It is purely a blob of data (i.e. normally "file |
||||
contents"). |
||||
|
||||
In particular, since the blob is entirely defined by its data, if two |
||||
files in a directory tree (or in multiple different versions of the |
||||
repository) have the same contents, they will share the same blob |
||||
object. The object is totally independent of its location in the |
||||
directory tree, and renaming a file does not change the object that |
||||
file is associated with in any way. |
||||
|
||||
A blob is typically created when gitlink:git-update-index[1] |
||||
(or gitlink:git-add[1]) is run, and its data can be accessed by |
||||
gitlink:git-cat-file[1]. |
||||
|
||||
Tree Object |
||||
~~~~~~~~~~~ |
||||
The next hierarchical object type is the "tree" object. A tree object |
||||
is a list of mode/name/blob data, sorted by name. Alternatively, the |
||||
mode data may specify a directory mode, in which case instead of |
||||
naming a blob, that name is associated with another TREE object. |
||||
|
||||
Like the "blob" object, a tree object is uniquely determined by the |
||||
set contents, and so two separate but identical trees will always |
||||
share the exact same object. This is true at all levels, i.e. it's |
||||
true for a "leaf" tree (which does not refer to any other trees, only |
||||
blobs) as well as for a whole subdirectory. |
||||
|
||||
For that reason a "tree" object is just a pure data abstraction: it |
||||
has no history, no signatures, no verification of validity, except |
||||
that since the contents are again protected by the hash itself, we can |
||||
trust that the tree is immutable and its contents never change. |
||||
|
||||
So you can trust the contents of a tree to be valid, the same way you |
||||
can trust the contents of a blob, but you don't know where those |
||||
contents 'came' from. |
||||
|
||||
Side note on trees: since a "tree" object is a sorted list of |
||||
"filename+content", you can create a diff between two trees without |
||||
actually having to unpack two trees. Just ignore all common parts, |
||||
and your diff will look right. In other words, you can effectively |
||||
(and efficiently) tell the difference between any two random trees by |
||||
O(n) where "n" is the size of the difference, rather than the size of |
||||
the tree. |
||||
|
||||
Side note 2 on trees: since the name of a "blob" depends entirely and |
||||
exclusively on its contents (i.e. there are no names or permissions |
||||
involved), you can see trivial renames or permission changes by |
||||
noticing that the blob stayed the same. However, renames with data |
||||
changes need a smarter "diff" implementation. |
||||
|
||||
A tree is created with gitlink:git-write-tree[1] and |
||||
its data can be accessed by gitlink:git-ls-tree[1]. |
||||
Two trees can be compared with gitlink:git-diff-tree[1]. |
||||
|
||||
Commit Object |
||||
~~~~~~~~~~~~~ |
||||
The "commit" object is an object that introduces the notion of |
||||
history into the picture. In contrast to the other objects, it |
||||
doesn't just describe the physical state of a tree, it describes how |
||||
we got there, and why. |
||||
|
||||
A "commit" is defined by the tree-object that it results in, the |
||||
parent commits (zero, one or more) that led up to that point, and a |
||||
comment on what happened. Again, a commit is not trusted per se: |
||||
the contents are well-defined and "safe" due to the cryptographically |
||||
strong signatures at all levels, but there is no reason to believe |
||||
that the tree is "good" or that the merge information makes sense. |
||||
The parents do not have to actually have any relationship with the |
||||
result, for example. |
||||
|
||||
Note on commits: unlike real SCM's, commits do not contain |
||||
rename information or file mode change information. All of that is |
||||
implicit in the trees involved (the result tree, and the result trees |
||||
of the parents), and describing that makes no sense in this idiotic |
||||
file manager. |
||||
|
||||
A commit is created with gitlink:git-commit-tree[1] and |
||||
its data can be accessed by gitlink:git-cat-file[1]. |
||||
|
||||
Trust |
||||
~~~~~ |
||||
An aside on the notion of "trust". Trust is really outside the scope |
||||
of "git", but it's worth noting a few things. First off, since |
||||
everything is hashed with SHA1, you 'can' trust that an object is |
||||
intact and has not been messed with by external sources. So the name |
||||
of an object uniquely identifies a known state - just not a state that |
||||
you may want to trust. |
||||
|
||||
Furthermore, since the SHA1 signature of a commit refers to the |
||||
SHA1 signatures of the tree it is associated with and the signatures |
||||
of the parent, a single named commit specifies uniquely a whole set |
||||
of history, with full contents. You can't later fake any step of the |
||||
way once you have the name of a commit. |
||||
|
||||
So to introduce some real trust in the system, the only thing you need |
||||
to do is to digitally sign just 'one' special note, which includes the |
||||
name of a top-level commit. Your digital signature shows others |
||||
that you trust that commit, and the immutability of the history of |
||||
commits tells others that they can trust the whole history. |
||||
|
||||
In other words, you can easily validate a whole archive by just |
||||
sending out a single email that tells the people the name (SHA1 hash) |
||||
of the top commit, and digitally sign that email using something |
||||
like GPG/PGP. |
||||
|
||||
To assist in this, git also provides the tag object... |
||||
|
||||
Tag Object |
||||
~~~~~~~~~~ |
||||
Git provides the "tag" object to simplify creating, managing and |
||||
exchanging symbolic and signed tokens. The "tag" object at its |
||||
simplest simply symbolically identifies another object by containing |
||||
the sha1, type and symbolic name. |
||||
|
||||
However it can optionally contain additional signature information |
||||
(which git doesn't care about as long as there's less than 8k of |
||||
it). This can then be verified externally to git. |
||||
|
||||
Note that despite the tag features, "git" itself only handles content |
||||
integrity; the trust framework (and signature provision and |
||||
verification) has to come from outside. |
||||
|
||||
A tag is created with gitlink:git-mktag[1], |
||||
its data can be accessed by gitlink:git-cat-file[1], |
||||
and the signature can be verified by |
||||
gitlink:git-verify-tag[1]. |
||||
|
||||
|
||||
The "index" aka "Current Directory Cache" |
||||
----------------------------------------- |
||||
The index is a simple binary file, which contains an efficient |
||||
representation of a virtual directory content at some random time. It |
||||
does so by a simple array that associates a set of names, dates, |
||||
permissions and content (aka "blob") objects together. The cache is |
||||
always kept ordered by name, and names are unique (with a few very |
||||
specific rules) at any point in time, but the cache has no long-term |
||||
meaning, and can be partially updated at any time. |
||||
|
||||
In particular, the index certainly does not need to be consistent with |
||||
the current directory contents (in fact, most operations will depend on |
||||
different ways to make the index 'not' be consistent with the directory |
||||
hierarchy), but it has three very important attributes: |
||||
|
||||
'(a) it can re-generate the full state it caches (not just the |
||||
directory structure: it contains pointers to the "blob" objects so |
||||
that it can regenerate the data too)' |
||||
|
||||
As a special case, there is a clear and unambiguous one-way mapping |
||||
from a current directory cache to a "tree object", which can be |
||||
efficiently created from just the current directory cache without |
||||
actually looking at any other data. So a directory cache at any one |
||||
time uniquely specifies one and only one "tree" object (but has |
||||
additional data to make it easy to match up that tree object with what |
||||
has happened in the directory) |
||||
|
||||
'(b) it has efficient methods for finding inconsistencies between that |
||||
cached state ("tree object waiting to be instantiated") and the |
||||
current state.' |
||||
|
||||
'(c) it can additionally efficiently represent information about merge |
||||
conflicts between different tree objects, allowing each pathname to be |
||||
associated with sufficient information about the trees involved that |
||||
you can create a three-way merge between them.' |
||||
|
||||
Those are the three ONLY things that the directory cache does. It's a |
||||
cache, and the normal operation is to re-generate it completely from a |
||||
known tree object, or update/compare it with a live tree that is being |
||||
developed. If you blow the directory cache away entirely, you generally |
||||
haven't lost any information as long as you have the name of the tree |
||||
that it described. |
||||
|
||||
At the same time, the index is at the same time also the |
||||
staging area for creating new trees, and creating a new tree always |
||||
involves a controlled modification of the index file. In particular, |
||||
the index file can have the representation of an intermediate tree that |
||||
has not yet been instantiated. So the index can be thought of as a |
||||
write-back cache, which can contain dirty information that has not yet |
||||
been written back to the backing store. |
||||
|
||||
|
||||
|
||||
The Workflow |
||||
------------ |
||||
Generally, all "git" operations work on the index file. Some operations |
||||
work *purely* on the index file (showing the current state of the |
||||
index), but most operations move data to and from the index file. Either |
||||
from the database or from the working directory. Thus there are four |
||||
main combinations: |
||||
|
||||
1) working directory -> index |
||||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ |
||||
|
||||
You update the index with information from the working directory with |
||||
the gitlink:git-update-index[1] command. You |
||||
generally update the index information by just specifying the filename |
||||
you want to update, like so: |
||||
|
||||
git-update-index filename |
||||
|
||||
but to avoid common mistakes with filename globbing etc, the command |
||||
will not normally add totally new entries or remove old entries, |
||||
i.e. it will normally just update existing cache entries. |
||||
|
||||
To tell git that yes, you really do realize that certain files no |
||||
longer exist, or that new files should be added, you |
||||
should use the `--remove` and `--add` flags respectively. |
||||
|
||||
NOTE! A `--remove` flag does 'not' mean that subsequent filenames will |
||||
necessarily be removed: if the files still exist in your directory |
||||
structure, the index will be updated with their new status, not |
||||
removed. The only thing `--remove` means is that update-cache will be |
||||
considering a removed file to be a valid thing, and if the file really |
||||
does not exist any more, it will update the index accordingly. |
||||
|
||||
As a special case, you can also do `git-update-index --refresh`, which |
||||
will refresh the "stat" information of each index to match the current |
||||
stat information. It will 'not' update the object status itself, and |
||||
it will only update the fields that are used to quickly test whether |
||||
an object still matches its old backing store object. |
||||
|
||||
2) index -> object database |
||||
~~~~~~~~~~~~~~~~~~~~~~~~~~~ |
||||
|
||||
You write your current index file to a "tree" object with the program |
||||
|
||||
git-write-tree |
||||
|
||||
that doesn't come with any options - it will just write out the |
||||
current index into the set of tree objects that describe that state, |
||||
and it will return the name of the resulting top-level tree. You can |
||||
use that tree to re-generate the index at any time by going in the |
||||
other direction: |
||||
|
||||
3) object database -> index |
||||
~~~~~~~~~~~~~~~~~~~~~~~~~~~ |
||||
|
||||
You read a "tree" file from the object database, and use that to |
||||
populate (and overwrite - don't do this if your index contains any |
||||
unsaved state that you might want to restore later!) your current |
||||
index. Normal operation is just |
||||
|
||||
git-read-tree <sha1 of tree> |
||||
|
||||
and your index file will now be equivalent to the tree that you saved |
||||
earlier. However, that is only your 'index' file: your working |
||||
directory contents have not been modified. |
||||
|
||||
4) index -> working directory |
||||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ |
||||
|
||||
You update your working directory from the index by "checking out" |
||||
files. This is not a very common operation, since normally you'd just |
||||
keep your files updated, and rather than write to your working |
||||
directory, you'd tell the index files about the changes in your |
||||
working directory (i.e. `git-update-index`). |
||||
|
||||
However, if you decide to jump to a new version, or check out somebody |
||||
else's version, or just restore a previous tree, you'd populate your |
||||
index file with read-tree, and then you need to check out the result |
||||
with |
||||
|
||||
git-checkout-index filename |
||||
|
||||
or, if you want to check out all of the index, use `-a`. |
||||
|
||||
NOTE! git-checkout-index normally refuses to overwrite old files, so |
||||
if you have an old version of the tree already checked out, you will |
||||
need to use the "-f" flag ('before' the "-a" flag or the filename) to |
||||
'force' the checkout. |
||||
|
||||
|
||||
Finally, there are a few odds and ends which are not purely moving |
||||
from one representation to the other: |
||||
|
||||
5) Tying it all together |
||||
~~~~~~~~~~~~~~~~~~~~~~~~ |
||||
To commit a tree you have instantiated with "git-write-tree", you'd |
||||
create a "commit" object that refers to that tree and the history |
||||
behind it - most notably the "parent" commits that preceded it in |
||||
history. |
||||
|
||||
Normally a "commit" has one parent: the previous state of the tree |
||||
before a certain change was made. However, sometimes it can have two |
||||
or more parent commits, in which case we call it a "merge", due to the |
||||
fact that such a commit brings together ("merges") two or more |
||||
previous states represented by other commits. |
||||
|
||||
In other words, while a "tree" represents a particular directory state |
||||
of a working directory, a "commit" represents that state in "time", |
||||
and explains how we got there. |
||||
|
||||
You create a commit object by giving it the tree that describes the |
||||
state at the time of the commit, and a list of parents: |
||||
|
||||
git-commit-tree <tree> -p <parent> [-p <parent2> ..] |
||||
|
||||
and then giving the reason for the commit on stdin (either through |
||||
redirection from a pipe or file, or by just typing it at the tty). |
||||
|
||||
git-commit-tree will return the name of the object that represents |
||||
that commit, and you should save it away for later use. Normally, |
||||
you'd commit a new `HEAD` state, and while git doesn't care where you |
||||
save the note about that state, in practice we tend to just write the |
||||
result to the file pointed at by `.git/HEAD`, so that we can always see |
||||
what the last committed state was. |
||||
|
||||
Here is an ASCII art by Jon Loeliger that illustrates how |
||||
various pieces fit together. |
||||
|
||||
------------ |
||||
|
||||
commit-tree |
||||
commit obj |
||||
+----+ |
||||
| | |
||||
| | |
||||
V V |
||||
+-----------+ |
||||
| Object DB | |
||||
| Backing | |
||||
| Store | |
||||
+-----------+ |
||||
^ |
||||
write-tree | | |
||||
tree obj | | |
||||
| | read-tree |
||||
| | tree obj |
||||
V |
||||
+-----------+ |
||||
| Index | |
||||
| "cache" | |
||||
+-----------+ |
||||
update-index ^ |
||||
blob obj | | |
||||
| | |
||||
checkout-index -u | | checkout-index |
||||
stat | | blob obj |
||||
V |
||||
+-----------+ |
||||
| Working | |
||||
| Directory | |
||||
+-----------+ |
||||
|
||||
------------ |
||||
|
||||
|
||||
6) Examining the data |
||||
~~~~~~~~~~~~~~~~~~~~~ |
||||
|
||||
You can examine the data represented in the object database and the |
||||
index with various helper tools. For every object, you can use |
||||
gitlink:git-cat-file[1] to examine details about the |
||||
object: |
||||
|
||||
git-cat-file -t <objectname> |
||||
|
||||
shows the type of the object, and once you have the type (which is |
||||
usually implicit in where you find the object), you can use |
||||
|
||||
git-cat-file blob|tree|commit|tag <objectname> |
||||
|
||||
to show its contents. NOTE! Trees have binary content, and as a result |
||||
there is a special helper for showing that content, called |
||||
`git-ls-tree`, which turns the binary content into a more easily |
||||
readable form. |
||||
|
||||
It's especially instructive to look at "commit" objects, since those |
||||
tend to be small and fairly self-explanatory. In particular, if you |
||||
follow the convention of having the top commit name in `.git/HEAD`, |
||||
you can do |
||||
|
||||
git-cat-file commit HEAD |
||||
|
||||
to see what the top commit was. |
||||
|
||||
7) Merging multiple trees |
||||
~~~~~~~~~~~~~~~~~~~~~~~~~ |
||||
|
||||
Git helps you do a three-way merge, which you can expand to n-way by |
||||
repeating the merge procedure arbitrary times until you finally |
||||
"commit" the state. The normal situation is that you'd only do one |
||||
three-way merge (two parents), and commit it, but if you like to, you |
||||
can do multiple parents in one go. |
||||
|
||||
To do a three-way merge, you need the two sets of "commit" objects |
||||
that you want to merge, use those to find the closest common parent (a |
||||
third "commit" object), and then use those commit objects to find the |
||||
state of the directory ("tree" object) at these points. |
||||
|
||||
To get the "base" for the merge, you first look up the common parent |
||||
of two commits with |
||||
|
||||
git-merge-base <commit1> <commit2> |
||||
|
||||
which will return you the commit they are both based on. You should |
||||
now look up the "tree" objects of those commits, which you can easily |
||||
do with (for example) |
||||
|
||||
git-cat-file commit <commitname> | head -1 |
||||
|
||||
since the tree object information is always the first line in a commit |
||||
object. |
||||
|
||||
Once you know the three trees you are going to merge (the one |
||||
"original" tree, aka the common case, and the two "result" trees, aka |
||||
the branches you want to merge), you do a "merge" read into the |
||||
index. This will complain if it has to throw away your old index contents, so you should |
||||
make sure that you've committed those - in fact you would normally |
||||
always do a merge against your last commit (which should thus match |
||||
what you have in your current index anyway). |
||||
|
||||
To do the merge, do |
||||
|
||||
git-read-tree -m -u <origtree> <yourtree> <targettree> |
||||
|
||||
which will do all trivial merge operations for you directly in the |
||||
index file, and you can just write the result out with |
||||
`git-write-tree`. |
||||
|
||||
Historical note. We did not have `-u` facility when this |
||||
section was first written, so we used to warn that |
||||
the merge is done in the index file, not in your |
||||
working tree, and your working tree will not match your |
||||
index after this step. |
||||
This is no longer true. The above command, thanks to `-u` |
||||
option, updates your working tree with the merge results for |
||||
paths that have been trivially merged. |
||||
|
||||
|
||||
8) Merging multiple trees, continued |
||||
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ |
||||
|
||||
Sadly, many merges aren't trivial. If there are files that have |
||||
been added, moved or removed, or if both branches have modified the |
||||
same file, you will be left with an index tree that contains "merge |
||||
entries" in it. Such an index tree can 'NOT' be written out to a tree |
||||
object, and you will have to resolve any such merge clashes using |
||||
other tools before you can write out the result. |
||||
|
||||
You can examine such index state with `git-ls-files --unmerged` |
||||
command. An example: |
||||
|
||||
------------------------------------------------ |
||||
$ git-read-tree -m $orig HEAD $target |
||||
$ git-ls-files --unmerged |
||||
100644 263414f423d0e4d70dae8fe53fa34614ff3e2860 1 hello.c |
||||
100644 06fa6a24256dc7e560efa5687fa84b51f0263c3a 2 hello.c |
||||
100644 cc44c73eb783565da5831b4d820c962954019b69 3 hello.c |
||||
------------------------------------------------ |
||||
|
||||
Each line of the `git-ls-files --unmerged` output begins with |
||||
the blob mode bits, blob SHA1, 'stage number', and the |
||||
filename. The 'stage number' is git's way to say which tree it |
||||
came from: stage 1 corresponds to `$orig` tree, stage 2 `HEAD` |
||||
tree, and stage3 `$target` tree. |
||||
|
||||
Earlier we said that trivial merges are done inside |
||||
`git-read-tree -m`. For example, if the file did not change |
||||
from `$orig` to `HEAD` nor `$target`, or if the file changed |
||||
from `$orig` to `HEAD` and `$orig` to `$target` the same way, |
||||
obviously the final outcome is what is in `HEAD`. What the |
||||
above example shows is that file `hello.c` was changed from |
||||
`$orig` to `HEAD` and `$orig` to `$target` in a different way. |
||||
You could resolve this by running your favorite 3-way merge |
||||
program, e.g. `diff3` or `merge`, on the blob objects from |
||||
these three stages yourself, like this: |
||||
|
||||
------------------------------------------------ |
||||
$ git-cat-file blob 263414f... >hello.c~1 |
||||
$ git-cat-file blob 06fa6a2... >hello.c~2 |
||||
$ git-cat-file blob cc44c73... >hello.c~3 |
||||
$ merge hello.c~2 hello.c~1 hello.c~3 |
||||
------------------------------------------------ |
||||
|
||||
This would leave the merge result in `hello.c~2` file, along |
||||
with conflict markers if there are conflicts. After verifying |
||||
the merge result makes sense, you can tell git what the final |
||||
merge result for this file is by: |
||||
|
||||
mv -f hello.c~2 hello.c |
||||
git-update-index hello.c |
||||
|
||||
When a path is in unmerged state, running `git-update-index` for |
||||
that path tells git to mark the path resolved. |
||||
|
||||
The above is the description of a git merge at the lowest level, |
||||
to help you understand what conceptually happens under the hood. |
||||
In practice, nobody, not even git itself, uses three `git-cat-file` |
||||
for this. There is `git-merge-index` program that extracts the |
||||
stages to temporary files and calls a "merge" script on it: |
||||
|
||||
git-merge-index git-merge-one-file hello.c |
||||
|
||||
and that is what higher level `git merge -s resolve` is implemented |
||||
with. |
@ -0,0 +1,161 @@
@@ -0,0 +1,161 @@
|
||||
Generating patches with -p |
||||
-------------------------- |
||||
|
||||
When "git-diff-index", "git-diff-tree", or "git-diff-files" are run |
||||
with a '-p' option, "git diff" without the '--raw' option, or |
||||
"git log" with the "-p" option, they |
||||
do not produce the output described above; instead they produce a |
||||
patch file. You can customize the creation of such patches via the |
||||
GIT_EXTERNAL_DIFF and the GIT_DIFF_OPTS environment variables. |
||||
|
||||
What the -p option produces is slightly different from the traditional |
||||
diff format. |
||||
|
||||
1. It is preceded with a "git diff" header, that looks like |
||||
this: |
||||
|
||||
diff --git a/file1 b/file2 |
||||
+ |
||||
The `a/` and `b/` filenames are the same unless rename/copy is |
||||
involved. Especially, even for a creation or a deletion, |
||||
`/dev/null` is _not_ used in place of `a/` or `b/` filenames. |
||||
+ |
||||
When rename/copy is involved, `file1` and `file2` show the |
||||
name of the source file of the rename/copy and the name of |
||||
the file that rename/copy produces, respectively. |
||||
|
||||
2. It is followed by one or more extended header lines: |
||||
|
||||
old mode <mode> |
||||
new mode <mode> |
||||
deleted file mode <mode> |
||||
new file mode <mode> |
||||
copy from <path> |
||||
copy to <path> |
||||
rename from <path> |
||||
rename to <path> |
||||
similarity index <number> |
||||
dissimilarity index <number> |
||||
index <hash>..<hash> <mode> |
||||
|
||||
3. TAB, LF, double quote and backslash characters in pathnames |
||||
are represented as `\t`, `\n`, `\"` and `\\`, respectively. |
||||
If there is need for such substitution then the whole |
||||
pathname is put in double quotes. |
||||
|
||||
The similarity index is the percentage of unchanged lines, and |
||||
the dissimilarity index is the percentage of changed lines. It |
||||
is a rounded down integer, followed by a percent sign. The |
||||
similarity index value of 100% is thus reserved for two equal |
||||
files, while 100% dissimilarity means that no line from the old |
||||
file made it into the new one. |
||||
|
||||
|
||||
combined diff format |
||||
-------------------- |
||||
|
||||
"git-diff-tree", "git-diff-files" and "git-diff" can take '-c' or |
||||
'--cc' option to produce 'combined diff'. For showing a merge commit |
||||
with "git log -p", this is the default format. |
||||
A 'combined diff' format looks like this: |
||||
|
||||
------------ |
||||
diff --combined describe.c |
||||
index fabadb8,cc95eb0..4866510 |
||||
--- a/describe.c |
||||
+++ b/describe.c |
||||
@@@ -98,20 -98,12 +98,20 @@@ |
||||
return (a_date > b_date) ? -1 : (a_date == b_date) ? 0 : 1; |
||||
} |
||||
|
||||
- static void describe(char *arg) |
||||
-static void describe(struct commit *cmit, int last_one) |
||||
++static void describe(char *arg, int last_one) |
||||
{ |
||||
+ unsigned char sha1[20]; |
||||
+ struct commit *cmit; |
||||
struct commit_list *list; |
||||
static int initialized = 0; |
||||
struct commit_name *n; |
||||
|
||||
+ if (get_sha1(arg, sha1) < 0) |
||||
+ usage(describe_usage); |
||||
+ cmit = lookup_commit_reference(sha1); |
||||
+ if (!cmit) |
||||
+ usage(describe_usage); |
||||
+ |
||||
if (!initialized) { |
||||
initialized = 1; |
||||
for_each_ref(get_name); |
||||
------------ |
||||
|
||||
1. It is preceded with a "git diff" header, that looks like |
||||
this (when '-c' option is used): |
||||
|
||||
diff --combined file |
||||
+ |
||||
or like this (when '--cc' option is used): |
||||
|
||||
diff --cc file |
||||
|
||||
2. It is followed by one or more extended header lines |
||||
(this example shows a merge with two parents): |
||||
|
||||
index <hash>,<hash>..<hash> |
||||
mode <mode>,<mode>..<mode> |
||||
new file mode <mode> |
||||
deleted file mode <mode>,<mode> |
||||
+ |
||||
The `mode <mode>,<mode>..<mode>` line appears only if at least one of |
||||
the <mode> is different from the rest. Extended headers with |
||||
information about detected contents movement (renames and |
||||
copying detection) are designed to work with diff of two |
||||
<tree-ish> and are not used by combined diff format. |
||||
|
||||
3. It is followed by two-line from-file/to-file header |
||||
|
||||
--- a/file |
||||
+++ b/file |
||||
+ |
||||
Similar to two-line header for traditional 'unified' diff |
||||
format, `/dev/null` is used to signal created or deleted |
||||
files. |
||||
|
||||
4. Chunk header format is modified to prevent people from |
||||
accidentally feeding it to `patch -p1`. Combined diff format |
||||
was created for review of merge commit changes, and was not |
||||
meant for apply. The change is similar to the change in the |
||||
extended 'index' header: |
||||
|
||||
@@@ <from-file-range> <from-file-range> <to-file-range> @@@ |
||||
+ |
||||
There are (number of parents + 1) `@` characters in the chunk |
||||
header for combined diff format. |
||||
|
||||
Unlike the traditional 'unified' diff format, which shows two |
||||
files A and B with a single column that has `-` (minus -- |
||||
appears in A but removed in B), `+` (plus -- missing in A but |
||||
added to B), or `" "` (space -- unchanged) prefix, this format |
||||
compares two or more files file1, file2,... with one file X, and |
||||
shows how X differs from each of fileN. One column for each of |
||||
fileN is prepended to the output line to note how X's line is |
||||
different from it. |
||||
|
||||
A `-` character in the column N means that the line appears in |
||||
fileN but it does not appear in the result. A `+` character |
||||
in the column N means that the line appears in the result, |
||||
and fileN does not have that line (in other words, the line was |
||||
added, from the point of view of that parent). |
||||
|
||||
In the above example output, the function signature was changed |
||||
from both files (hence two `-` removals from both file1 and |
||||
file2, plus `++` to mean one line that was added does not appear |
||||
in either file1 nor file2). Also eight other lines are the same |
||||
from file1 but do not appear in file2 (hence prefixed with `{plus}`). |
||||
|
||||
When shown by `git diff-tree -c`, it compares the parents of a |
||||
merge commit with the merge result (i.e. file1..fileN are the |
||||
parents). When shown by `git diff-files -c`, it compares the |
||||
two unresolved merge parents with the working tree file |
||||
(i.e. file1 is stage 2 aka "our version", file2 is stage 3 aka |
||||
"their version"). |
Some files were not shown because too many files have changed in this diff Show More
Loading…
Reference in new issue