Browse Source
The libfdt functions are supposed to behave tolerably well when practical, even if given a corrupted device tree as input. A silly mistake in fdt_get_property() means we're bounds checking against the size of a pointer instead of the size of a property header, meaning we can get bogus behaviour in a corrupted device tree where the structure block ends in what's supposed to be the middle of a property. This patch corrects the problem (fdt_get_property() will now return BADSTRUCTURE in this case), and also adds a testcase to catch the bug.main
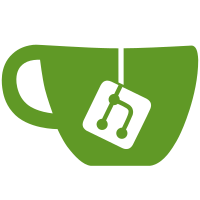
6 changed files with 72 additions and 2 deletions
@ -0,0 +1,49 @@
@@ -0,0 +1,49 @@
|
||||
/* |
||||
* libfdt - Flat Device Tree manipulation |
||||
* Testcase for misbehaviour on a truncated property |
||||
* Copyright (C) 2006 David Gibson, IBM Corporation. |
||||
* |
||||
* This library is free software; you can redistribute it and/or |
||||
* modify it under the terms of the GNU Lesser General Public License |
||||
* as published by the Free Software Foundation; either version 2.1 of |
||||
* the License, or (at your option) any later version. |
||||
* |
||||
* This library is distributed in the hope that it will be useful, but |
||||
* WITHOUT ANY WARRANTY; without even the implied warranty of |
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU |
||||
* Lesser General Public License for more details. |
||||
* |
||||
* You should have received a copy of the GNU Lesser General Public |
||||
* License along with this library; if not, write to the Free Software |
||||
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA |
||||
*/ |
||||
|
||||
#include <stdlib.h> |
||||
#include <stdio.h> |
||||
#include <string.h> |
||||
|
||||
#include <fdt.h> |
||||
#include <libfdt.h> |
||||
|
||||
#include "tests.h" |
||||
#include "testdata.h" |
||||
|
||||
int main(int argc, char *argv[]) |
||||
{ |
||||
void *fdt = &_truncated_property; |
||||
void *prop; |
||||
int err; |
||||
int len; |
||||
|
||||
test_init(argc, argv); |
||||
|
||||
prop = fdt_getprop(fdt, 0, "truncated", &len); |
||||
err = fdt_ptr_error(prop); |
||||
if (! err) |
||||
FAIL("fdt_getprop() succeeded on truncated property"); |
||||
if (err != FDT_ERR_BADSTRUCTURE) |
||||
FAIL("fdt_getprop() failed with \"%s\" instead of \"%s\"", |
||||
fdt_strerror(err), fdt_strerror(FDT_ERR_BADSTRUCTURE)); |
||||
|
||||
PASS(); |
||||
} |
Loading…
Reference in new issue