Browse Source
Given a device tree node, a property name and an index, the new function fdt_stringlist_get() will return a pointer to the index'th string in the property's value and return its length (or an error code on failure) in an output argument. Signed-off-by: Thierry Reding <treding@nvidia.com> [Fix some -Wshadow warnings --dwg] Signed-off-by: David Gibson <david@gibson.dropbear.id.au>main
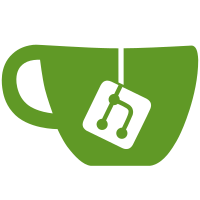
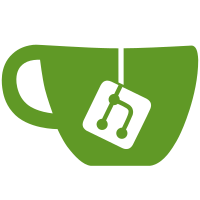
3 changed files with 105 additions and 0 deletions
Loading…
Reference in new issue