Browse Source
some HW has different flavors of basic libs $ ldconfig -p|fgrep libc.so libc.so.6 (libc6,64bit, hwcap: 0x0000001000000000, OS ABI: Linux 2.6.32) => /lib64/power6/libc.so.6 libc.so.6 (libc6,64bit, hwcap: 0x0000000000000200, OS ABI: Linux 2.6.32) => /lib64/power6x/libc.so.6 libc.so.6 (libc6,64bit, OS ABI: Linux 2.6.32) => /lib64/libc.so.6 because setting LD_HWCAP_MASK=0 does not work, we have to workaround this. $ LD_TRACE_LOADED_OBJECTS=1 LD_HWCAP_MASK=0 /lib64/ld64.so.1 /bin/sh | fgrep libc.so libc.so.6 => /lib64/power6/libc.so.6 (0x000000804e260000) Now we try to install the same library from one directory above the one we installed also.master
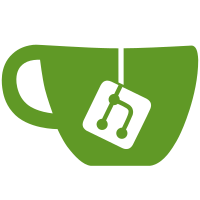
3 changed files with 164 additions and 19 deletions
Loading…
Reference in new issue